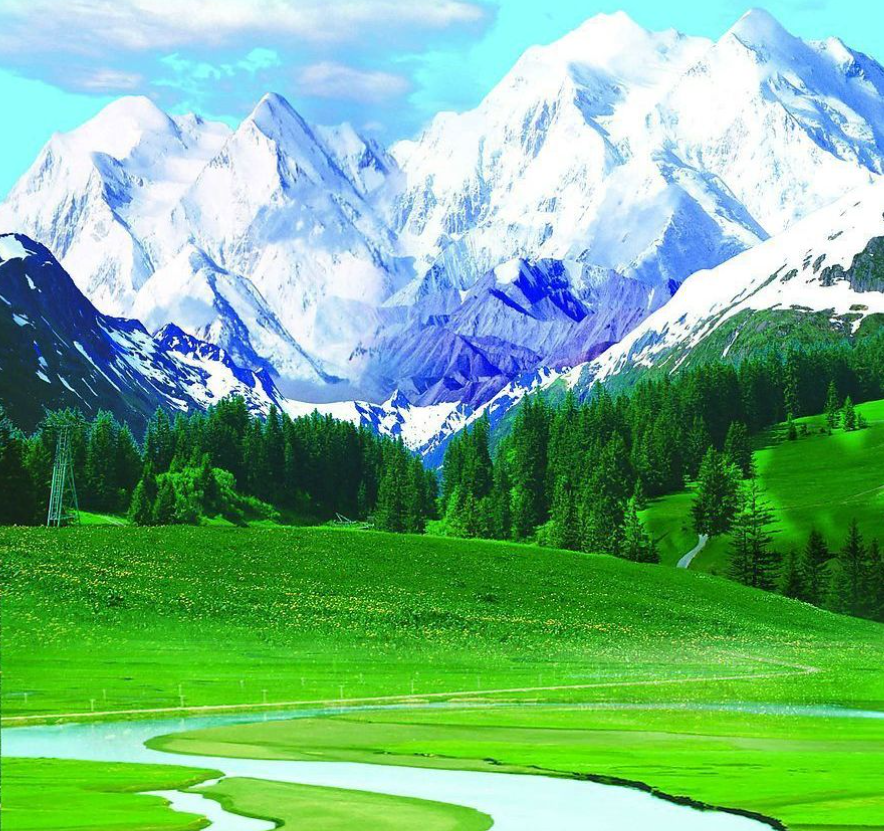
Python中的字典该怎么用,看这一篇就够了(结尾有惊喜)
目录1.基本概念2.常用操作(1)使用字典1.基本形式2.访问字典中的值3.添加(或创建)键值对4.修改字典中的值5.删除键值对(2)遍历字典1.遍历字典中所有的键值对2.遍历字典的所有键3.遍历字典中的所有值(3)嵌套1.字典列表2.在字典中存储列表3.在字典中存储字典(4)结尾小彩蛋1.基本概念在python中,字典是一系列的键-值对,可用于存储需要一一对应的数据。 字典是一系列的键-值对,键
目录
1.基本概念
在python中,字典是一系列的键-值对,可用于存储需要一一对应的数据。 字典是一系列的键-值对,键和值之间用冒号隔开,而键-值对之间用逗号隔开。 代码示例如下: 字典的键一般是唯一的,如果出现重复,最后的一个键值对会替换前面的。
2.常用操作
(1)使用字典
1.基本形式
school={'student':'xiaoming','score':90}
2.访问字典中的值
方法 | 描述 |
---|---|
key | 使用键作为下标访问元素,如果键不存在,那么程序会抛出异常。 |
get() | 使用 get() 方法访问元素,如果键不存在,那么程序会不会抛出异常,而是返回一个空值,同时,该方法可以设置键不存在时,使用的默认值。 |
items() | 使用 items() 访问字典,返回是一个 KEY 对应一个 VALUE 的元祖的列表的形式。 |
keys() | 使用 keys() 访问字典,返回的是字典的所有的 KEY。 |
values() | 使用 values() 访问字典,返回的是字典的所有的 VALUE。 |
school={'student':'xiaoming','score':90}
print(school["score"])
print(school.get('student','NO point value assigned'))
print(school.get('aaa','NO point value assigned'))
print(school.items())
print(school.keys())
print(school.values())
运行结果:
90
xiaoming
NO point value assigned
dict_items([('student', 'xiaoming'), ('score', 90)])
dict_keys(['student', 'score'])
dict_values(['xiaoming', 90])
3.添加(或创建)键值对
直接对字典中不存在的key进行赋值来添加
school={'student':'xiaoming','score':90}
school['stunumber']=209
print(school)
运行结果:
{'student': 'xiaoming', 'score': 90, 'stunumber': 209}
如果key或value都是变量也可以用这种方法
dic={}
key='age'
values=30
dic[key]=values
print(dic)
运行结果:
{'age': 30}
还可以用字典的setdefault方法
dic={}
dic.setdefault('sex','male')
print(dic)
key='id'
value='001'
dic.setdefault(key,value)
print(dic)
运行结果:
{'sex': 'male'}
{'sex': 'male', 'id': '001'}
4.修改字典中的值
在确定key值存在的情况下,直接根据指定的键修改
school={'student':'xiaoming','score':90}
school['student']='dahong'
print(school)
运行结果:
{'student': 'dahong', 'score': 90}
在不确定key值是否存在的情况下,
使用字典(Dictionary
)的TryGetValue()
方法来判断指定键是否存在
int val;
if (dic.TryGetValue(key, out val))
{
//如果指定的字典的键存在
dic[key] = newValue;
}
else
{
//不存在,则添加
dic.Add(key, newValue);
}
还可以使用LINQ
来访问字典的键并修改对应的值
Dictionary<string, int> dict = new Dictionary<string, int>();
dict = dict.ToDictionary(x => x.Key, x => x.Value + 1);
5.删除键值对
clear()方法(删除字典内所有元素)
school={'student':'xiaoming','score':90}
school.clear()
print(school)
运行结果:
{}
pop()方法(删除字典给定键 key 所对应的值,返回值为被删除的值)
school={'student':'xiaoming','score':90}
school.pop('student')
print(school)
运行结果:
{'score': 90}
popitem()方法(删除字典最后的一对键和值)
school={'student':'xiaoming','score':90}
print(school.popitem())
运行结果:
('score', 90)
del 全局方法(能删单一的元素也能清空字典,清空只需一项操作)
school={'student':'xiaoming','score':90}
del school['student']
print(school)
aaa={'bbb':'xiaoming','ccc':90}
del aaa
print(aaa)
运行结果:
{'score': 90}
Traceback (most recent call last):
File "D:\pycharm\pythonProject\venv\678.py", line 1783, in <module>
print(aaa)
NameError: name 'aaa' is not defined
(2)遍历字典
1.遍历字典中所有的键值对
school={'student':'xiaoming','score':90}
for key,value in school.items():
print("\nKey:"+key)
print("value:"+str(value))
运行结果:
Key:student
value:xiaomingKey:score
value:90
2.遍历字典的所有键
school={'student':'xiaoming','score':90}
for key in school.keys():
print(key)
运行结果:
student
score
3.遍历字典中的所有值
school={'student':'xiaoming','score':90}
for value in school.values():
print(value)
运行结果:
xiaoming
90
(3)嵌套
1.字典列表
student_1={'stunumber':204,'score':90}
student_2={'stunumber':205,'score':94}
student_3={'stunumber':206,'score':80}
students=[student_1,student_2,student_3]
print(students)
运行结果:
[{'stunumber': 204, 'score': 90}, {'stunumber': 205, 'score': 94}, {'stunumber': 206, 'score': 80}]
2.在字典中存储列表
# 建立一个存储披萨信息的字典
pizza = {
'crust': 'thick',
# 字典中包含两个元素的列表
'toppings': ['mushrooms', 'extra cheese']
}
print(f"You ordered a {pizza['crust']}-crust pizza"
" with the following toppings:")
for topping in pizza['toppings']:
print('\t'+ topping)
运行结果:
You ordered a thick-crust pizza with the following toppings:
mushrooms
extra cheese
3.在字典中存储字典
users={
'aeinstein':{
'first':'albert',
'last':'einstein',
'location':'princeton',
},
'mcurie':{
'first':'marie',
'last':'curie',
'location':'paris',
},
}
for username,user_info in users.items():
print(f"\nUsername:{username}")
full_name=f"{user_info['first']} {user_info['last']}"
location=user_info['location']
print(f"\tFull name:{full_name.title()}")
print(f"\tLocation :{location.title()}")
运行结果:
Username:aeinstein
Full name:Albert Einstein
Location :PrincetonUsername:mcurie
Full name:Marie Curie
Location :Paris
(4)结尾小彩蛋
最后再和大家总结一波python常用内置函数
函数名 | 作用 |
---|---|
print() | 打印输出 |
input() | 获取用户输出内容 |
open() | 用于打开一个文件,创建一个文件句柄 |
list() | 将一个可迭代对象转化为列表 |
tuple() | 将一个可迭代对象转化为元组 |
reversed() | 将一个序列反转,返回反转序列的迭代器 |
range() | 生成数据 |
str() | 将数据转化为字符串 |
all() | 可迭代对象中全部是True,结果才是True |
any() | 可迭代对象有一个是True,结果就是True |
eval() | 执行字符串类型的代码,并返回最终结果 |
dir() | 查看对象的内置属性,访问的是对象的_dir_()方法 |
help() | 函数用于查看函数或模块用途的仔细说明 |
更多推荐
所有评论(0)