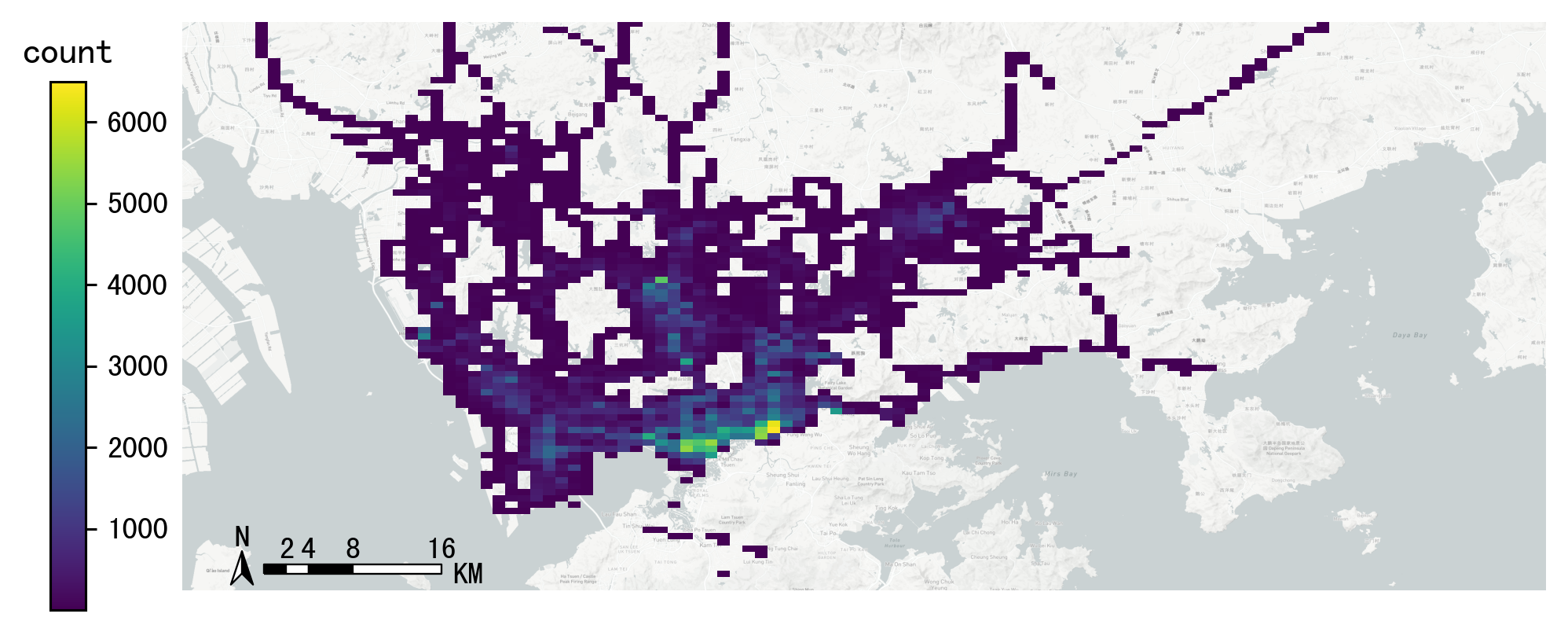
Python实现geohash编码与解码(TransBigData)
geohash编码geohash是一种公共域地理编码系统,它的作用是将经纬度地理位置编码为字母和数字组成的字符串,字符串也可解码为经纬度。每个字符串代表一个网格编号,字符串的长度越长则精度越高。根据wiki,geohash字符串长度对应精度表格如下:geohash length(precision)lat bitslng bitslat errorlng errorkm
geohash编码
geohash是一种公共域地理编码系统,它的作用是将经纬度地理位置编码为字母和数字组成的字符串,字符串也可解码为经纬度。每个字符串代表一个网格编号,字符串的长度越长则精度越高。根据wiki,geohash字符串长度对应精度表格如下:
geohash length(precision) | lat bits | lng bits | lat error | lng error | km error |
---|---|---|---|---|---|
1 | 2 | 3 | ±23 | ±23 | ±2500 |
2 | 5 | 5 | ±2.8 | ±5.6 | ±630 |
3 | 7 | 8 | ±0.70 | ±0.70 | ±78 |
4 | 10 | 10 | ±0.087 | ±0.18 | ±20 |
5 | 12 | 13 | ±0.022 | ±0.022 | ±2.4 |
6 | 15 | 15 | ±0.0027 | ±0.0055 | ±0.61 |
7 | 17 | 18 | ±0.00068 | ±0.00068 | ±0.076 |
8 | 20 | 20 | ±0.000085 | ±0.00017 | ±0.019 |
Python的TransBigData包中提供了geohash的处理功能,主要包括三个函数:
- transbigdata.geohash_encode(lon, lat, precision=12)
输入经纬度与精度,输出geohash编码
- transbigdata.geohash_decode(geohash)
输入geohash编码,输出经纬度
- transbigdata.geohash_togrid(geohash)
输入geohash编码,输出geohash网格的地理信息图形Series列
相比TransBigData包中提供的方形栅格处理方法,geohash更慢,也无法提供自由定义的栅格大小。下面的示例展示如何利用这三个函数对数据进行geohash编码集计,并可视化
import transbigdata as tbd
import pandas as pd
import geopandas as gpd
#读取数据
data = pd.read_csv('TaxiData-Sample.csv',header = None)
data.columns = ['VehicleNum','time','slon','slat','OpenStatus','Speed']
#依据经纬度geohash编码,精确度选6时,栅格大小约为±0.61km
data['geohash'] = tbd.geohash_encode(data['slon'],data['slat'],precision=6)
data['geohash']
0 ws0btw 1 ws0btz 2 ws0btz 3 ws0btz 4 ws0by4 ... 544994 ws131q 544995 ws1313 544996 ws131f 544997 ws1361 544998 ws10tq Name: geohash, Length: 544999, dtype: object
#基于geohash编码集计
dataagg = data.groupby(['geohash'])['VehicleNum'].count().reset_index()
#geohash编码解码为经纬度
dataagg['lon_geohash'],dataagg['lat_geohash'] = tbd.geohash_decode(dataagg['geohash'])
#geohash编码生成栅格矢量图形
dataagg['geometry'] = tbd.geohash_togrid(dataagg['geohash'])
#转换为GeoDataFrame
dataagg = gpd.GeoDataFrame(dataagg)
dataagg
geohash | VehicleNum | lon_geohash | lat_geohash | geometry | |
---|---|---|---|---|---|
0 | w3uf3x | 1 | 108. | 10.28 | POLYGON ((107.99561 10.27771, 107.99561 10.283... |
1 | webzz6 | 12 | 113.9 | 22.47 | POLYGON ((113.87329 22.46704, 113.87329 22.472... |
2 | webzz7 | 21 | 113.9 | 22.48 | POLYGON ((113.87329 22.47253, 113.87329 22.478... |
3 | webzzd | 1 | 113.9 | 22.47 | POLYGON ((113.88428 22.46704, 113.88428 22.472... |
4 | webzzf | 2 | 113.9 | 22.47 | POLYGON ((113.89526 22.46704, 113.89526 22.472... |
... | ... | ... | ... | ... | ... |
2022 | ws1d9u | 1 | 114.7 | 22.96 | POLYGON ((114.68628 22.96143, 114.68628 22.966... |
2023 | ws1ddh | 6 | 114.7 | 22.96 | POLYGON ((114.69727 22.96143, 114.69727 22.966... |
2024 | ws1ddj | 2 | 114.7 | 22.97 | POLYGON ((114.69727 22.96692, 114.69727 22.972... |
2025 | ws1ddm | 4 | 114.7 | 22.97 | POLYGON ((114.70825 22.96692, 114.70825 22.972... |
2026 | ws1ddq | 7 | 114.7 | 22.98 | POLYGON ((114.70825 22.97241, 114.70825 22.977... |
2027 rows × 5 columns
#设定绘图边界
bounds = [113.6,22.4,114.8,22.9]
#创建图框
import matplotlib.pyplot as plt
import plot_map
fig =plt.figure(1,(8,8),dpi=280)
ax =plt.subplot(111)
plt.sca(ax)
#添加地图底图
tbd.plot_map(plt,bounds,zoom = 12,style = 4)
#绘制colorbar
cax = plt.axes([0.05, 0.33, 0.02, 0.3])
plt.title('count')
plt.sca(ax)
#绘制geohash的栅格集计
dataagg.plot(ax = ax,column = 'VehicleNum',cax = cax,legend = True)
#添加比例尺和指北针
tbd.plotscale(ax,bounds = bounds,textsize = 10,compasssize = 1,accuracy = 2000,rect = [0.06,0.03],zorder = 10)
plt.axis('off')
plt.xlim(bounds[0],bounds[2])
plt.ylim(bounds[1],bounds[3])
plt.show()
更多推荐
所有评论(0)