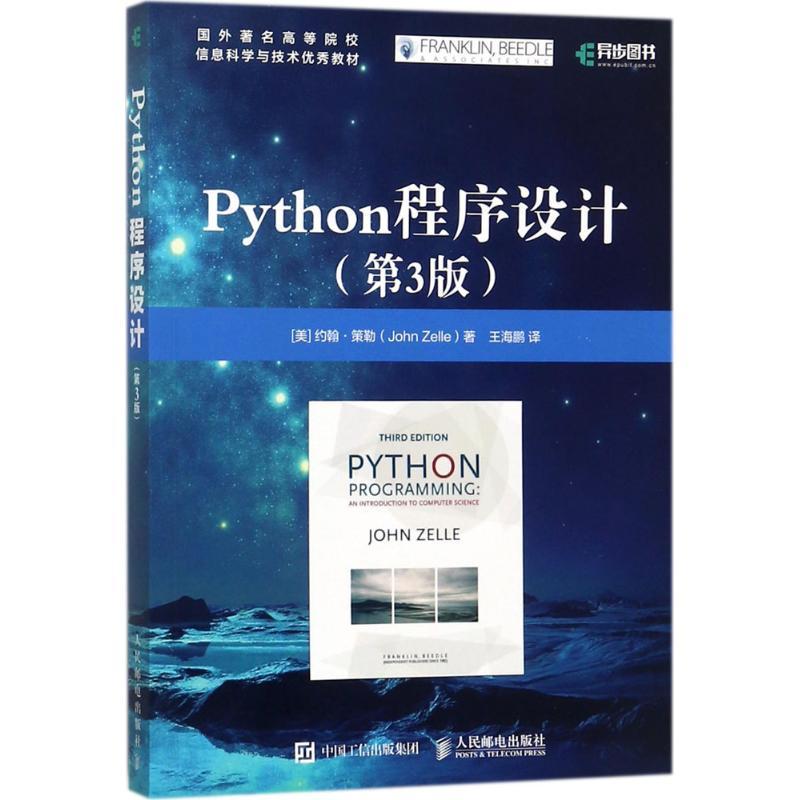
《 Python程序设计(第3版)》[美] 约翰·策勒(John Zelle) 第 8 章 答案
True/FalseFalseTrueFalseTrueFalseFalseTrueTrueFalseTrueMultiple ChoiceacdcccdbcaDiscussionWhile loop fragments:sum = 0i = 1while i <= n:sum = sum + ii = i + 1sum = 0i = 1while .
·
True/False
- False
- True
- False
- True
- False
- False
- True
- True
- False
- True
Multiple Choice
- a
- c
- d
- c
- c
- c
- d
- b
- c
- a
Discussion
- While loop fragments:
-
sum = 0 i = 1 while i <= n: sum = sum + i i = i + 1
-
sum = 0 i = 1 while i < 2*n: sum = sum + i i = i + 2
-
sum = 0 x = input("Enter a number: ") while x != 999: sum = sum + x x = input("Enter a number: ")
-
count = 0 while n > 1: n = n / 2 count = count + 1
-
Programming Exercises
1.
解法一
def main():
a = 0
b = 1
c = 1
n = int(input("Enter n: "))
# for i in range(n-1):
i = 0
while i < n - 1:
i = i + 1
c = a + b
a = b
b = c
print("The figure is {0}.".format(c))
main()
解法二
# c08ex01.py
# Nth fibonacci number
def main():
print("This program calculates the nth Fibonacci value.\n")
n = int(input("Enter the value of n: "))
curr, prev = 1, 1
for i in range(n-2):
curr, prev = curr+prev, curr
print("The nth Fibonacci number is", curr)
if __name__ == '__main__':
main()
2.
解法一(没有用好函数和列表,缺乏简洁性)
def drawStruct():
print('\n|----------------+',end = '')
for i in range(8):
print('-'*8+'+',end = '')
print('--------|')
def drawLine():
print("|{0:^16}|".format(' '),end = '')
for i in range(9):
print("{0:^8}|".format(' '),end = '')
print()
def main():
print('WCI'.center(100))
print('-'*99)
print("|{0:^15}|".format('V/ML/h \ T/℃'),end = '')
for T in range(-20,61,10):
print("{0:^8}|".format(T),end = '')
drawStruct()
for V in range(0,55,5):
drawLine()
print("|{0:^16}|".format(V),end = '')
for T in range(-20,61,10):
if V <= 3:
WCI = '/'
print("{0:^8}|".format(WCI),end = '')
else:
WCI = 35.74 + 0.6215 * T + (0.4275 * T - 35.75) * (V ** 0.16)
print("{0:^8.2f}|".format(WCI),end = '')
print()
print('-'*99)
if __name__ =="__main__" :
main()
解法二(最佳解法,值得学习)
# c08ex02.py
# Windchill Table
def windChill(t, v):
if v > 3:
chill = 35.74 + 0.6215*t - 35.75*(v**0.16) + 0.4275*t*(v**0.16)
else:
chill = t
return chill
def main():
# Print table headings
print("Wind Chill Table\n\n")
print("Temperature".center(70))
print("MPH|", end=' ')
temps = list(range(-20,61,10))
for t in temps:
print("{0:5}".format(t), end=' ')
print("\n---+" + 55 * '-')
# Print lines in table
for vel in range(0,51,5):
print("{0:3}|".format(vel), end=' ')
for temp in temps:
print("{0:5.0f}".format(windChill(temp, vel)), end=' ')
print()
print()
main()
3.
def main():
apr = float(input("Enter the interest: "))
n = 0
while (1+apr)**n < 2:
n = n + 1
print('Principal will double after {0} years.'.format(n))
if __name__ == "__main__":
main()
4
def main():
n = int(input('Enter n: '))
print('Syracuse:',n,end = ' ')
while n != 1:
n = syr(n)
print(int(n),end = ' ')
def syr(x):
if x % 2==0:s = x/2
else:s = 3 * x + 1
return s
if __name__ =='__main__':
main()
5
解法一(更简洁易懂)
def main():
n = int(input('Enter n(n>2): '))
s = 0
for i in range(2,n):
if n % i == 0:
print(i,end = ' ')
s = s + 1
if s == 0:
print(n,'is prime.')
if __name__ == "__main__":
main()
解法二(算法结构更老道)
# c08ex05.py
# Primality tester
import math
def isPrime(n):
if n % 2 == 0:
return False
factor = 3
while factor <= math.sqrt(n):
if n % factor == 0:
return False
factor = factor + 2
return True
def main():
print("Prime number tester\n")
n = int(input("Enter a value to test: "))
if isPrime(n):
print(n, "is prime.")
else:
print(n, "is not prime.")
if __name__ == '__main__':
main()
6
解法一
def main():
n = int(input('Enter n(n>2): '))
for j in range(2,n + 1):
isPrime(j)
def isPrime(n):
s = 0
for i in range(2,n):
if n % i == 0:
s = s + 1
if s == 0:
print(n,end = ' ')
if __name__ == "__main__":
main()
解法二
# c08ex06.py
# primes to n
from c08ex05 import isPrime
def main():
print("This program finds all prime numbers up to N\n")
n = int(input("Enter the upper limit, n: "))
print("primes: ", end=' ')
for i in range(2,n+1):
if isPrime(i):
print(i, end=' ')
print("Done")
if __name__ == '__main__':
main()
7
解法一
def main():
try:
n = int(input('Enter n: '))
t = 0
if n % 2 == 0:
m = n // 2
for i in range(2,m):
if isPrime(i) + isPrime(n - i) == 2:
t = t + 1
print('({0}) {1} {2}'.format(t,i,n-i))
else: print('The number is not an even number!')
except:
print('Check the figure you input!')
def isPrime(n):
s = 0
for i in range(2,n):
if n % i == 0:
s = s + 1
if s == 0: return 1
else: return 0
if __name__ == '__main__':
main()
解法二
# c08ex07.py
# Goldbach tester
from c08ex05 import isPrime
def goldbach(x):
cand = 3
while cand < x/2:
other = x - cand
if isPrime(cand) and isPrime(other):
return cand
cand = cand + 2
def main():
print("Goldbach checker\n")
n = int(input("Enter an even natural number: "))
if n % 2 != 0:
print(n, "is not even!")
else:
prime1 = goldbach(n)
prime2 = n - prime1
print("{0} + {1} = {2}".format(prime1, prime2, n))
if __name__ == '__main__':
main()
8.
def main():
m = int(input('Enter m: '))
n = int(input('Enter n: '))
while m !=0:
n , m = m , n % m
print('GCD:',n)
if __name__ == '__main__':
main()
9
解法一
def main():
startRoute = float(input('Enter the route at first: '))
totalRoute = 0
totalGas = 0
i = 0
while True:
rg = input('Enter the route and gas seperated by " ":').split()
if rg == []:
break
i = i + 1
route,gas = float(rg[0]),float(rg[1])
MPG = (route - startRoute) / gas
totalRoute = totalRoute + route - startRoute
startRoute = route
totalGas = totalGas + gas
print('Route{0} MPG: {1:.2f}.'.format(i,MPG))
totalMPG = totalRoute / totalGas
print('Total MPG: {0:.2f}'.format(totalMPG))
if __name__ == '__main__':
main()
解法二
# c08ex09.py
# Program to figure out gas mileage overa multiple-leg journey.
def main():
print("This program calculates fuel efficiency over a multi-leg journey.")
print("You should enter the gallons of gas consumed and miles traveled")
print("for each leg. Just hit <Enter> to signal the end of the trip.")
print()
distance, fuel = 0.0, 0.0
inStr = input("Enter gallons and miles (with a comma between): ")
while inStr != "":
inputs = inStr.split(",")
gallons, miles = int(inputs[0]), int(inputs[1])
print("MPG for this leg: {0:0.1f}".format(miles/gallons))
distance = distance + miles
fuel = fuel + gallons
inStr = input("Enter gallons and miles (with a comma between): ")
print()
print("You traveled a total of {0:0.1f} miles on {1:0.1f} gallons."
.format(distance, fuel))
print("The fuel efficiency was {0:0.1f} miles per gallon."
.format(distance/fuel))
if __name__ == '__main__':
main()
10
解法一
def main():
fname = input('Enter the name of the file: ')
infile = open(fname,'r',encoding = 'utf-8')
startRoute = float(infile.readline())
totalRoute = 0
totalGas = 0
i = 0
for line in infile.readlines():
rg = line.split()
if rg == []:
break
i = i + 1
route,gas = float(rg[0]),float(rg[1])
MPG = (route - startRoute) / gas
totalRoute = totalRoute + route - startRoute
startRoute = route
totalGas = totalGas + gas
print('Route{0} MPG: {1:.2f}.'.format(i,MPG))
totalMPG = totalRoute / totalGas
print('Total MPG: {0:.2f}'.format(totalMPG))
infile.close()
if __name__ == '__main__':
main()
解法二
# c08ex10.py
# Batch fuel efficiency
def main():
print("This program calculates fuel efficiency over a multi-leg journey.")
print("It gets trip information from a file. Each line of the file should")
print("have the gallons used and miles traveled (separated by a comma) for")
print("one leg of the journey.\n")
fname = input("What file contains the data? ")
infile = open(fname,'r')
distance, fuel = 0.0, 0.0
for line in infile:
inputs = line.split(",")
gallons, miles = int(inputs[0]), int(inputs[1])
print("Gallons: {0:0.1f}, Miles: {1:0.1f}, MPG: {2:0.1f}"
.format(gallons, miles, miles/gallons))
distance = distance + miles
fuel = fuel + gallons
infile.close()
print("\nYou traveled a total of {0:0.1f} miles on {1:0.1f} gallons."
.format(distance, fuel))
print("The fuel efficiency was {0:0.1f} miles per gallon."
.format(distance/fuel))
if __name__ == '__main__':
main()
11
解法一
def main():
i,hot,cold = 1,0,0
while True:
temperature = input('Enter the average temperature of day{0}: '.format(i))
if temperature == '':break
t = float(temperature)
if t < 60:hot = hot + 60 - t
if t > 80:cold = cold + t - 80
i = i + 1
print('Cooling degree-days:{0} Heating degree-days:{1}'.format(cold,hot))
if __name__ == '__main__':
main()
解法二(使用max语句替代if语句,更简洁,经验值得积累)
# c08ex11.py
# heating/colling degree-days
def main():
print("Heating and Cooling degree-day calculation.\n")
heating = 0.0
cooling = 0.0
tempStr = input("Enter an average daily temperature: ")
while tempStr != "":
temp = float(tempStr)
heating = heating + max(0, 60-temp)
cooling = cooling + max(0, temp-80)
tempStr = input("Enter an average daily temperature: ")
print("\nTotal heating degree-days", heating)
print("Total cooling degree-days", cooling)
if __name__ == '__main__':
main()
12
def main():
print("Heating and Cooling degree-day calculation.")
fname = input("Enter name of file with average temperature data: ")
infile = open(fname, 'r')
heating = 0.0
cooling = 0.0
for line in infile:
temp = float(line)
heating = heating + max(0, 60-temp)
cooling = cooling + max(0, temp-80)
infile.close()
print("\nTotal heating degree-days", heating)
print("Total cooling degree-days", cooling)
if __name__ == '__main__':
main()
13
解法一
from graphics import *
def main():
win = GraphWin('线性回归方程演示',700,700)
win.setBackground('white')
win.setCoords(0,0,50,50)
frame = Rectangle(Point(0,0),Point(4,2))
frame.setFill(color_rgb(0,255,144))
frame.draw(win)
Text(Point(2,1),'Done').draw(win)
sxy,sx,sy,sx2,sy2,n = 0,0,0,0,0,0
while True:
p = win.getMouse()
px,py = p.getX(),p.getY()
if px <= 4 and py <= 2:
break
p.draw(win)
sxy = sxy + px * py
sx2 = sx2 + px**2
sy2 = sy2 + py**2
sx = sx + px
sy = sy + py
n = n + 1
a = Circle(Point(sx/n,sy/n),.30)
a.setFill('red')
a.setOutline('red')
a.setWidth(2)
a.draw(win)
m = (sxy - sx*sy/n)/(sx2 - sx*sx/n)
n = (sy - sx*m)/n
l = Line(Point(0,n),Point(50,m*50 + n))
l.setWidth(2)
l.draw(win)
win.getMouse()
win.close()
if __name__ =='__main__':
main()
解法二
from graphics import *
# These two "constants" are defined here so they can be used in multiple
# functions to draw the done button and check to see if it is clicked in.
DoneX = 1.0 # x coordinate of done button upper right
DoneY = 0.75 # y coordinate of done button upper right
def createWindow():
# Creates a 500x500 window titled "Linear Regression" and draws
# a "Done Button" in the lower left corner. The window coords
# run from 0,0 to 10,10, and the upper right corner of the button
# is located at (DoneX, DoneY).
# Returns the window.
win = GraphWin("Linear Regression", 500, 500)
win.setCoords(0,0,10,10)
doneButton = Rectangle(Point(.05,0), Point(DoneX, DoneY))
doneButton.draw(win)
Text(doneButton.getCenter(), "Done").draw(win)
return win
def getPoint(w):
# w is a GraphWin
# Pauses for user to click in w. If user clicks somewhere other than
# the Done button, the point is drawn in w.
# Returns the point clicked, or None if user clicked the Done button
p = w.getMouse()
if p.getX() > DoneX or p.getY() > DoneY:
p.draw(w)
return p
else:
return None
def slope(xb, yb, xx, xy, n):
# Returns the slope of the line of best fit, m
return (xy - n * xb * yb) / (xx - n*xb*xb)
def predict(x, xb, yb, m):
# Returns a predicated value for y.
return yb + m*(x-xb)
def getPoints(w):
# Allows the user to make a series of mouse clicks in w until
# the user clicks in the Done button.
# Returns: average of x values
# average of y values
# sum of the squares of the x values
# sum of the xy products
# the number of points clicked before Done
sumX = 0.0
sumY = 0.0
sumXsq = 0.0
sumXY = 0.0
n = 0
p = getPoint(w)
while p != None:
x,y = p.getX(), p.getY()
sumX = sumX + x
sumY = sumY + y
sumXY = sumXY + x * y
sumXsq = sumXsq + x * x
n = n + 1
p = getPoint(w)
return sumX/n, sumY/n, sumXsq, sumXY, n
def main():
# Draws a window, gets mouse clicks and then draws line of best
# fit through the points that were clicked.
win = createWindow()
xbar, ybar, sumXsq, sumXY, n = getPoints(win)
m = slope(xbar, ybar, sumXsq, sumXY, n)
y1 = predict(0, xbar, ybar, m)
y2 = predict(10, xbar, ybar, m)
regLine = Line(Point(0,y1), Point(10,y2))
regLine.setWidth(2)
regLine.draw(win)
win.getMouse()
win.close()
if __name__ == '__main__':
main()
14 这是一道很好的题,Image对象很少练习。这道题可做典型。
from graphics import *
def openImage(picFile):
img = Image(Point(0,0),picFile)
width = img.getWidth()
height = img.getHeight()
win = GraphWin('',width,height)
img.move(width//2,height//2)
img.draw(win)
return img
def convertToGray(img):
for row in range(img.getWidth()):
for col in range(img.getHeight()):
r,g,b = img.getPixel(row,col)
i = round(0.299*r + 0.587*g + 0.114*b)
img.setPixel(row,col,color_rgb(i,i,i))
update()
def main():
print("This program converts an image to grayscale.")
inFile = input("Enter the name of the gif file to convert: ")
image = openImage(inFile)
print('Convertin...',end = '')
convertToGray(image)
print('Done.')
outFile = input('Enter filename for the converted image: ')
image.save(outFile)
if __name__ =='__main__':
main()
15
from graphics import *
def openFile(picFile):
img = Image(Point(0,0),picFile)
width,height = img.getWidth(),img.getHeight()
win = GraphWin('',width,height)
img.move(width//2,height//2)
img.draw(win)
return img
def convertColor(img):
for row in range(img.getWidth()):
for col in range(img.getHeight()):
r,g,b = img.getPixel(row,col)
img.setPixel(row,col,color_rgb(255-r,255-g,255-b))
update()
def main():
picFile = input("Enter the name of file to be converted: ")
img = openFile(picFile)
convertColor(img)
outFile = input("Enter the name of the converted file: ")
img.save(outFile)
if __name__ == '__main__':
main()
16 有一个疑问,已经放在注释里了。日后精通python了,要解决!
from graphics import *
def handleKey(k,win):
if k == 'p':
win.setBackground('pink')
elif k == 'w':
win.setBackground('white')
elif k == 'g':
win.setBackground('lightgray')
elif k == 'b':
win.setBackground('lightblue')
elif k == 'y':
win.setBackground('yellow')
elif k == 'r':
win.setBackground('red')
def handleClick(pt,win):
entry = Entry(pt,10)
entry.draw(win)
while True:
key = win.getKey()
if key in ['Return','Escape']:break
# We cannot use "if key is == 'Return' or 'Escape'"
# to replace" if key in ['Return','Escape']",but why?
entry.undraw()
typed = entry.getText()
if key == 'Return':
Text(pt,typed).draw(win)
win.checkMouse()
def main():
win = GraphWin('Click and Type',500,500)
while True:
key = win.checkKey()
if key == 'q':
break
if key:
handleKey(key,win)
pt = win.checkMouse()
if pt:
handleClick(pt,win)
win.close()
main()
更多推荐
所有评论(0)