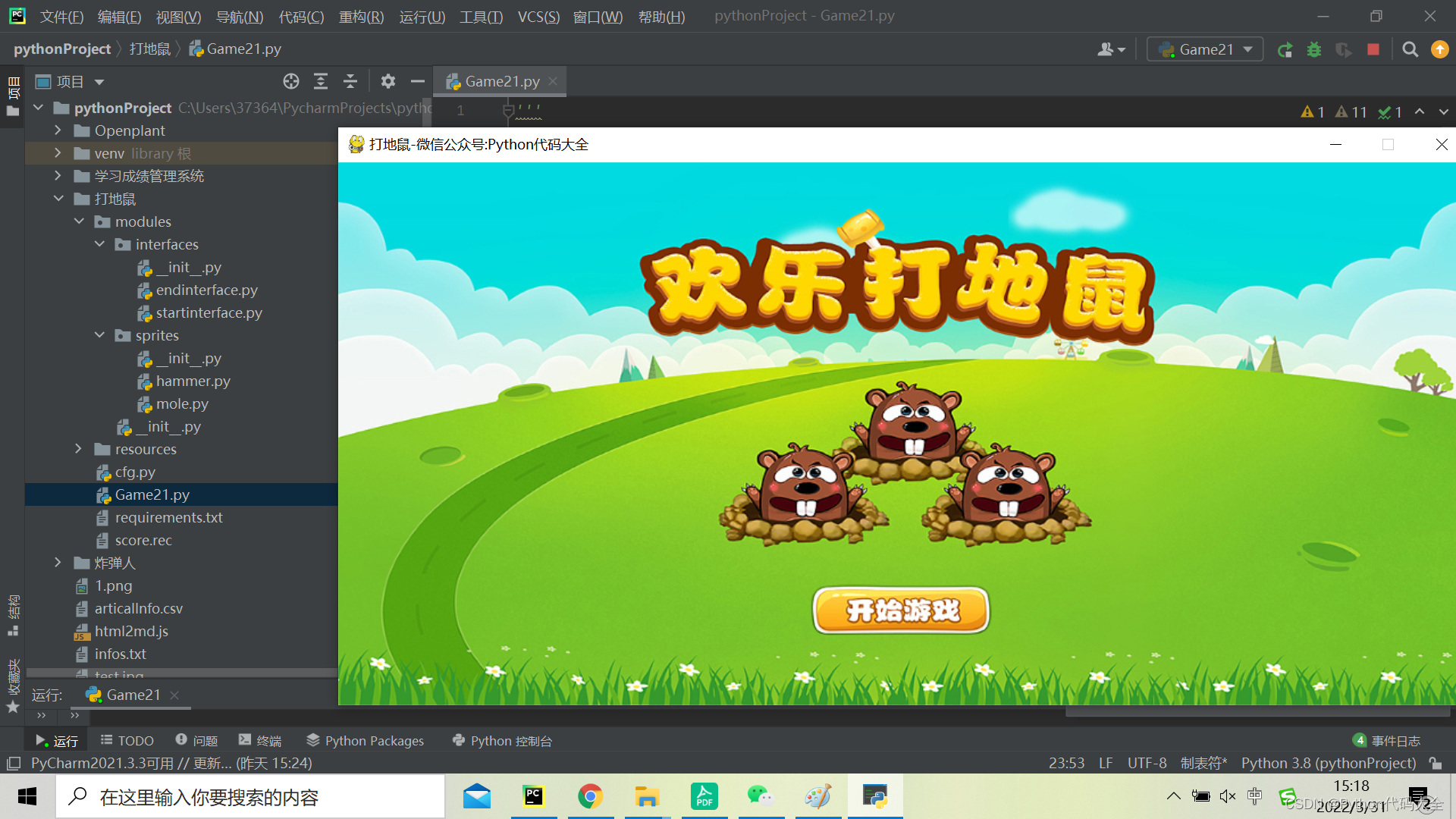
Python打地鼠小游戏源代码
Python打地鼠小游戏源代码,打地鼠游戏是一款具有童年回忆的游戏,不知你是否还记得童年时站在电玩城打地鼠游戏机的前面疯狂的挥动手中的棒槌,一直不停的打地鼠,那个时候是不是觉得很好玩呢。
·
Python打地鼠小游戏源代码,打地鼠游戏是一款具有童年回忆的游戏,不知你是否还记得童年时站在电玩城打地鼠游戏机的前面疯狂的挥动手中的棒槌,一直不停的打地鼠,那个时候是不是觉得很好玩呢。程序运行截图:
核心代码:
'''
Function:
打地鼠(Whac-A-Mole)小游戏
Author:
Charles
微信公众号:
Python代码大全
'''
import cfg
import pygame
import random
from modules.sprites.mole import *
from modules.sprites.hammer import *
from modules.interfaces.endinterface import *
from modules.interfaces.startinterface import *
'''游戏初始化'''
def initGame():
pygame.init()
pygame.mixer.init()
screen = pygame.display.set_mode(cfg.SCREENSIZE)
pygame.display.set_caption('打地鼠-微信公众号:Python代码大全')
return screen
'''主函数'''
def main():
# 初始化
screen = initGame()
# 加载背景音乐和其他音效
pygame.mixer.music.load(cfg.BGM_PATH)
pygame.mixer.music.play(-1)
audios = {
'count_down': pygame.mixer.Sound(cfg.COUNT_DOWN_SOUND_PATH),
'hammering': pygame.mixer.Sound(cfg.HAMMERING_SOUND_PATH)
}
# 加载字体
font = pygame.font.Font(cfg.FONT_PATH, 40)
# 加载背景图片
bg_img = pygame.image.load(cfg.GAME_BG_IMAGEPATH)
# 开始界面
startInterface(screen, cfg.GAME_BEGIN_IMAGEPATHS)
# 地鼠改变位置的计时
hole_pos = random.choice(cfg.HOLE_POSITIONS)
change_hole_event = pygame.USEREVENT
pygame.time.set_timer(change_hole_event, 800)
# 地鼠
mole = Mole(cfg.MOLE_IMAGEPATHS, hole_pos)
# 锤子
hammer = Hammer(cfg.HAMMER_IMAGEPATHS, (500, 250))
# 时钟
clock = pygame.time.Clock()
# 分数
your_score = 0
flag = False
# 初始时间
init_time = pygame.time.get_ticks()
# 游戏主循环
while True:
# --游戏时间为60s
time_remain = round((61000 - (pygame.time.get_ticks() - init_time)) / 1000.)
# --游戏时间减少, 地鼠变位置速度变快
if time_remain == 40 and not flag:
hole_pos = random.choice(cfg.HOLE_POSITIONS)
mole.reset()
mole.setPosition(hole_pos)
pygame.time.set_timer(change_hole_event, 650)
flag = True
elif time_remain == 20 and flag:
hole_pos = random.choice(cfg.HOLE_POSITIONS)
mole.reset()
mole.setPosition(hole_pos)
pygame.time.set_timer(change_hole_event, 500)
flag = False
# --倒计时音效
if time_remain == 10:
audios['count_down'].play()
# --游戏结束
if time_remain < 0: break
count_down_text = font.render('Time: '+str(time_remain), True, cfg.WHITE)
# --按键检测
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEMOTION:
hammer.setPosition(pygame.mouse.get_pos())
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
hammer.setHammering()
elif event.type == change_hole_event:
hole_pos = random.choice(cfg.HOLE_POSITIONS)
mole.reset()
mole.setPosition(hole_pos)
# --碰撞检测
if hammer.is_hammering and not mole.is_hammer:
is_hammer = pygame.sprite.collide_mask(hammer, mole)
if is_hammer:
audios['hammering'].play()
mole.setBeHammered()
your_score += 10
# --分数
your_score_text = font.render('Score: '+str(your_score), True, cfg.BROWN)
# --绑定必要的游戏元素到屏幕(注意顺序)
screen.blit(bg_img, (0, 0))
screen.blit(count_down_text, (875, 8))
screen.blit(your_score_text, (800, 430))
mole.draw(screen)
hammer.draw(screen)
# --更新
pygame.display.flip()
clock.tick(60)
# 读取最佳分数(try块避免第一次游戏无.rec文件)
try:
best_score = int(open(cfg.RECORD_PATH).read())
except:
best_score = 0
# 若当前分数大于最佳分数则更新最佳分数
if your_score > best_score:
f = open(cfg.RECORD_PATH, 'w')
f.write(str(your_score))
f.close()
# 结束界面
score_info = {'your_score': your_score, 'best_score': best_score}
is_restart = endInterface(screen, cfg.GAME_END_IMAGEPATH, cfg.GAME_AGAIN_IMAGEPATHS, score_info, cfg.FONT_PATH, [cfg.WHITE, cfg.RED], cfg.SCREENSIZE)
return is_restart
'''run'''
if __name__ == '__main__':
while True:
is_restart = main()
if not is_restart:
break
cfg.py
'''配置文件'''
import os
CURPATH = os.getcwd()
SCREENSIZE = (993, 477)
HAMMER_IMAGEPATHS = [os.path.join(CURPATH, 'resources/images/hammer0.png'), os.path.join(CURPATH, 'resources/images/hammer1.png')]
GAME_BEGIN_IMAGEPATHS = [os.path.join(CURPATH, 'resources/images/begin.png'), os.path.join(CURPATH, 'resources/images/begin1.png')]
GAME_AGAIN_IMAGEPATHS = [os.path.join(CURPATH, 'resources/images/again1.png'), os.path.join(CURPATH, 'resources/images/again2.png')]
GAME_BG_IMAGEPATH = os.path.join(CURPATH, 'resources/images/background.png')
GAME_END_IMAGEPATH = os.path.join(CURPATH, 'resources/images/end.png')
MOLE_IMAGEPATHS = [os.path.join(CURPATH, 'resources/images/mole_1.png'), os.path.join(CURPATH, 'resources/images/mole_laugh1.png'),
os.path.join(CURPATH, 'resources/images/mole_laugh2.png'), os.path.join(CURPATH, 'resources/images/mole_laugh3.png')]
HOLE_POSITIONS = [(90, -20), (405, -20), (720, -20), (90, 140), (405, 140), (720, 140), (90, 290), (405, 290), (720, 290)]
BGM_PATH = 'resources/audios/bgm.mp3'
COUNT_DOWN_SOUND_PATH = 'resources/audios/count_down.wav'
HAMMERING_SOUND_PATH = 'resources/audios/hammering.wav'
FONT_PATH = 'resources/font/Gabriola.ttf'
BROWN = (150, 75, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
RECORD_PATH = 'score.rec'
hammer.py
'''
Function:
定义锤子
Author:
Charles
微信公众号:
Python代码大全
'''
import pygame
'''锤子类'''
class Hammer(pygame.sprite.Sprite):
def __init__(self, image_paths, position, **kwargs):
pygame.sprite.Sprite.__init__(self)
self.images = [pygame.image.load(image_paths[0]), pygame.image.load(image_paths[1])]
self.image = self.images[0]
self.rect = self.image.get_rect()
self.mask = pygame.mask.from_surface(self.images[1])
self.rect.left, self.rect.top = position
# 用于显示锤击时的特效
self.hammer_count = 0
self.hammer_last_time = 4
self.is_hammering = False
'''设置位置'''
def setPosition(self, pos):
self.rect.centerx, self.rect.centery = pos
'''设置hammering'''
def setHammering(self):
self.is_hammering = True
'''显示在屏幕上'''
def draw(self, screen):
if self.is_hammering:
self.image = self.images[1]
self.hammer_count += 1
if self.hammer_count > self.hammer_last_time:
self.is_hammering = False
self.hammer_count = 0
else:
self.image = self.images[0]
screen.blit(self.image, self.rect)
完整程序代码下载:Python打地鼠小游戏源代码
更多Python源代码,请关注公众号:Python代码大全。
更多推荐
所有评论(0)