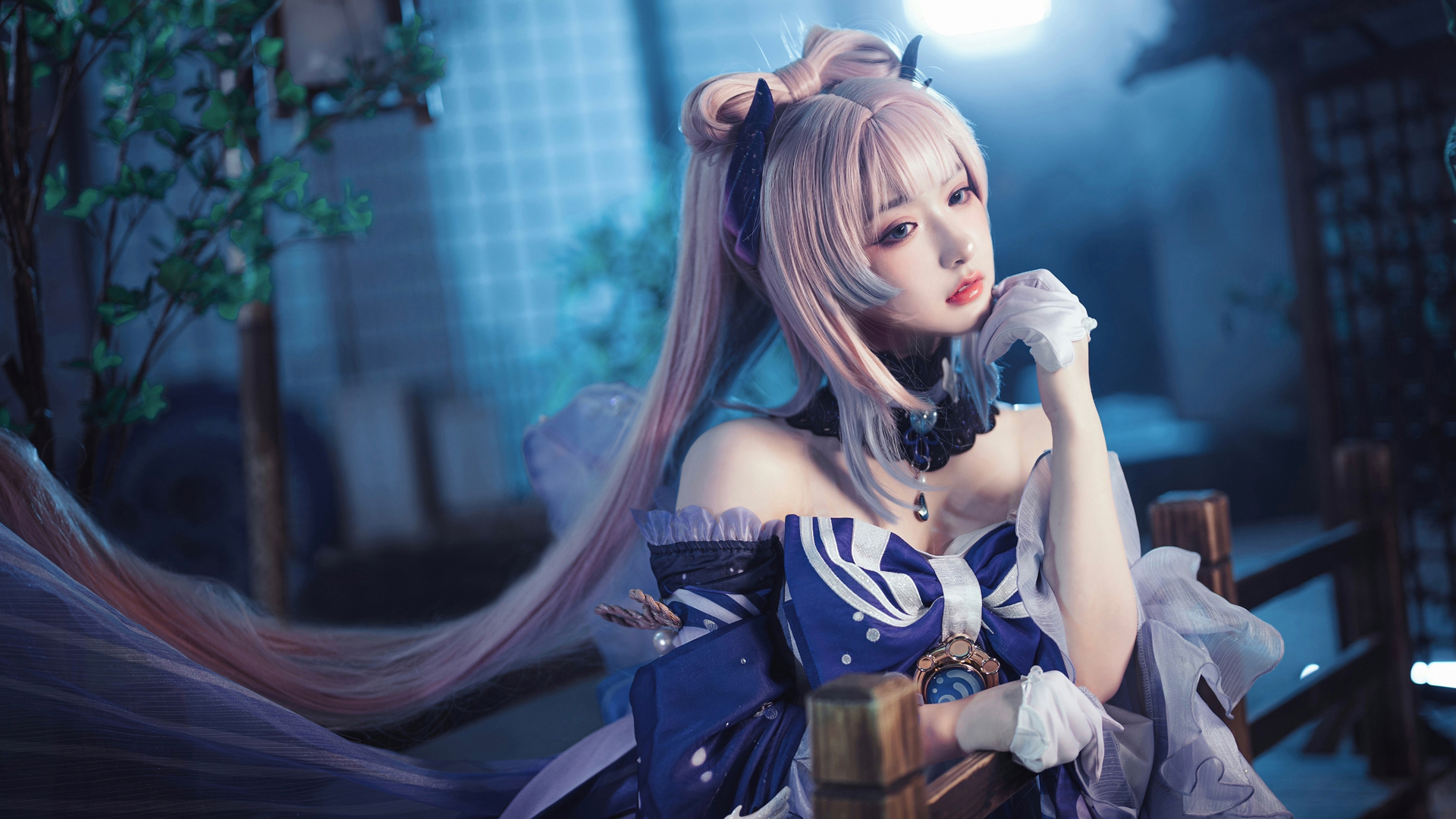
(娱乐项目)Python图片转换成矩阵数据,矩阵数据转换成图片
(娱乐项目)Python图片转换成矩阵数据,矩阵数据转换成图片
·
吴恩达机器学习的课程作业里,经常会出现以mat数据存储的图像,为此我十分好奇,中间过程是怎么实现的,这篇博客简单的学习一下:
首先,我们看一下图片:(图片来源于网络,如有侵权,请立刻联系我删除)
导入需要用到的包
import PIL.Image as Image
import numpy as np
import pandas as pd
import os
import matplotlib.pyplot as plt
from scipy.io import loadmat
file_name = "113936HmJVY.jpg"
将图片转换为矩阵
def image_to_matrix(file_name):
# 读取图片
image = Image.open(file_name)
# 显示图片
image.show()
width, height = image.size
# 灰度化
image_grey = image.convert("L")
data = image_grey.getdata()
data = np.matrix(data, dtype="float") / 255.0
new_data = np.reshape(data, (height, width))
return new_data
我们生成的数据矩阵为:
[[0.05098039 0.07058824 0.08235294 ... 0.11372549 0.10588235 0.11372549]
[0.05490196 0.07058824 0.09019608 ... 0.10588235 0.10588235 0.10980392]
[0.07058824 0.0745098 0.09411765 ... 0.10980392 0.10980392 0.10980392]
...
[0.07843137 0.09019608 0.08627451 ... 0.23529412 0.23921569 0.23137255]
[0.0627451 0.07058824 0.07058824 ... 0.23921569 0.23529412 0.22352941]
[0.07843137 0.0745098 0.0745098 ... 0.24705882 0.23921569 0.22745098]]
我们转换成dataframe的格式,方便查看:
dataframe = pd.DataFrame(data=data)
print(dataframe)
0 1 2 ... 1917 1918 1919
0 0.050980 0.070588 0.082353 ... 0.113725 0.105882 0.113725
1 0.054902 0.070588 0.090196 ... 0.105882 0.105882 0.109804
2 0.070588 0.074510 0.094118 ... 0.109804 0.109804 0.109804
3 0.066667 0.090196 0.125490 ... 0.109804 0.101961 0.105882
4 0.070588 0.109804 0.137255 ... 0.109804 0.101961 0.109804
... ... ... ... ... ... ... ...
1075 0.074510 0.062745 0.070588 ... 0.227451 0.227451 0.227451
1076 0.094118 0.094118 0.094118 ... 0.231373 0.239216 0.231373
1077 0.078431 0.090196 0.086275 ... 0.235294 0.239216 0.231373
1078 0.062745 0.070588 0.070588 ... 0.239216 0.235294 0.223529
1079 0.078431 0.074510 0.074510 ... 0.247059 0.239216 0.227451
可以看出,我们的图像大小为:1920*1080
将矩阵转换为图片
def matrix_to_image(data):
data = data * 255.0
new_im = Image.fromarray(data)
return new_im
new_im = matrix_to_image(data)
plt.imshow(data, cmap=plt.cm.gray, interpolation="nearest")
new_im.show()
可以实现!
上面要先对图片去除颜色,就是变成黑白的,转换成二维数据矩阵,不去颜色的还要保存颜色的,然后后面转换就不行了。然后利用Image.fromarray(data) 新建图片
完整代码
import PIL.Image as Image
import numpy as np
import pandas as pd
import os
import matplotlib.pyplot as plt
from scipy.io import loadmat
# 将图片转换为矩阵
def image_to_matrix(file_name):
# 读取图片
image = Image.open(file_name)
# 显示图片
image.show()
width, height = image.size
# 灰度化
image_grey = image.convert("L")
data = image_grey.getdata()
data = np.matrix(data, dtype="float") / 255.0
new_data = np.reshape(data, (height, width))
return new_data
# 将矩阵转换为图片
def matrix_to_image(data):
data = data * 255.0
new_im = Image.fromarray(data)
return new_im
# 定义图片路径
file_name = "113936HmJVY.jpg"
# 将图片转换为矩阵并输出到csv文件
data = image_to_matrix(file_name)
print(data)
print('=='*30)
dataframe = pd.DataFrame(data=data)
print(dataframe)
# dataframe.to_csv('d:/out.csv', sep=' ', header=False, float_format='%.2f', index=False)
# 将矩阵转换为图片
new_im = matrix_to_image(data)
plt.imshow(data, cmap=plt.cm.gray, interpolation="nearest")
new_im.show()
更多推荐
所有评论(0)