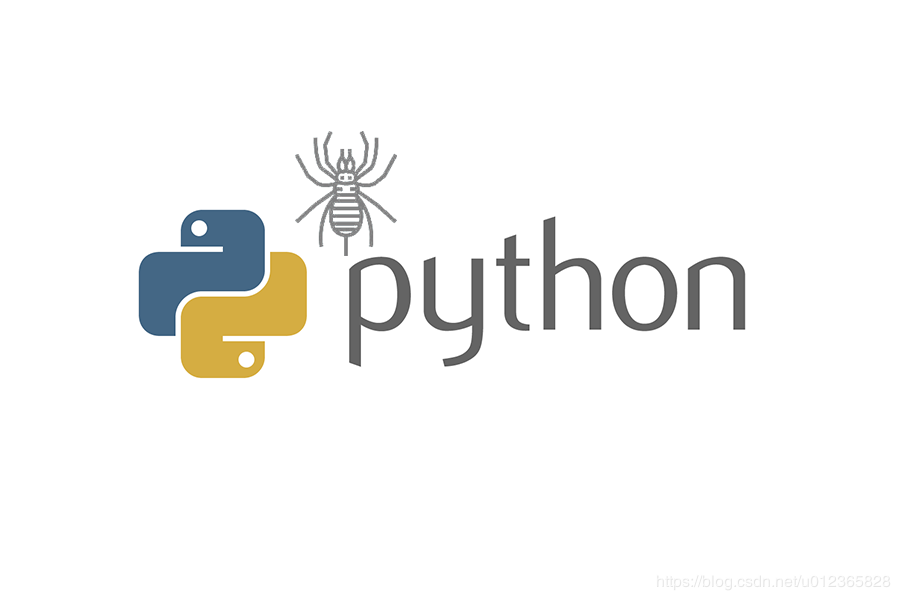
Python字典练习题
初始字典如下:dic = {"k1": "v1", "k2": "v2", "k3": "v3"}完成以下练习题目#?遍历字典 dic 中所有的keyfor ele in dic.keys():print(ele)#?b.遍历字典 dic 中所有的valuefor ele in dic.values():print(ele)#?c.循环遍历字典 dic 中所有的key和valuefor k,v i
·
初始字典如下:
dic = {"k1": "v1", "k2": "v2", "k3": "v3"}
完成以下练习题目
#?遍历字典 dic 中所有的key
for ele in dic.keys():
print(ele)
#?b.遍历字典 dic 中所有的value
for ele in dic.values():
print(ele)
#?c.循环遍历字典 dic 中所有的key和value
for k,v in dic.items():
print(k,v)
#?添加一个键值对"k4","v4",输出添加后的字典 dic
dic["k4"] = "v4"
print(dic)
#?e.删除字典 dic 中的键值对"k1","v1",并输出删除后的字典 dic
del dic["k1"]
print(dic)
#?f.删除字典 dic 中 'k5' 对应的值,若不存在,使其不报错,并返回None
if "K5" in ele:
del dic["k5"]
else:
print(dic.get("k5"))
#?g.获取字典 dic 中“k2”对应的值
print(dic["k2"])
print(dic.get("k2","0.0"))
新增一个字典如下:使得dic2 = {'k1':"v111",'k2':"v2",'k3':"v3",'k4': 'v4','a':"b"}
dic2 = {'k1':"v111",'a':"b"}
#两种方法
# ? 使用update()
dic2= {'k1':"v111",'a':"b"}
dic2.update({'k1':"v111",'k2':"v2",'k3':"v3",'k4': 'v4','a':"b"})
print(dic2)
# ?使用items()
dic = {'k2': 'v2', 'k3': 'v3', 'k4': 'v4'}
dic2 = {'k1':"v111",'a':"b"}
del dic2["a"]
#ele_list = list(dic2.keys())
for k,v in dic.items():
dic2[k] = v
dic2["a"] = "b"
print(dic2)
2.已知列表list1 = [11,22,11,33,44,55,66,55,66],
统计列表中每个元素出现的次数,生成一个字典,结果为{11:2,22:1.....}
list1 = [11,22,11,33,44,55,66,55,66]
new_dic = {}
for ele in list1:
new_dic[ele] = list1.count(ele)
print(new_dic)
3.已知如下列表students,在列表中保存了6个学生的信息,根据要求完成下面的题目
students = [
{'name': '小花', 'age': 19, 'score': 90, 'gender': '女', 'tel':
'15300022839'},
{'name': '明明', 'age': 20, 'score': 40, 'gender': '男', 'tel':
'15300022838'},
{'name': '华仔', 'age': 18, 'score': 90, 'gender': '女', 'tel':
'15300022839'},
{'name': '静静', 'age': 16, 'score': 90, 'gender': '不明', 'tel':
'15300022428'},
{'name': 'Tom', 'age': 17, 'score': 59, 'gender': '不明', 'tel':
'15300022839'},
{'name': 'Bob', 'age': 18, 'score': 90, 'gender': '男', 'tel':
'15300022839'}
]
使用循环知识点,以及建立变量名的规范
#? 统计不及格学生的个数
fail_stu = 0
for ele in students:
if ele["score"] <= 60:
fail_stu +=1
print(fail_stu)
#? b.打印不及格学生的名字和对应的成绩
stu_dic = {}
for ele in students:
if ele["score"] <= 60:
stu_dic[ele["name"]] = ele["score"]
print(stu_dic)
#? c.统计未成年学生的个数
minor_stu = 0
for ele in students:
if ele["age"] < 18:
minor_stu +=1
print(minor_stu)
#? d.打印手机尾号是8的学生的名字
for ele in students:
if ele["tel"][-1] == "8":
print(ele["name"])
#? e.打印最高分和对应的学生的名字
maxscore_stu =[]
stu_score = {}
max_score = 0
for ele in students:
maxscore_stu.append(ele["score"])
max_score = max(maxscore_stu)
for ele in students:
if ele["score"] == max_score:
stu_score[ele["name"]] = max_score
print(stu_score)
#? f.删除性别不明的所有学生
new_dic = []
for ele in students:
if ele["gender"] != "不明":
new_dic.append(ele)
print(new_dic)
#? g.将列表按学生成绩从大到小排序
students.sort(key = lambda x:x["score"])
print(students)
4.使用列表推导式完成下面的题目
#? a. 生成一个存放1-100之间个位数为3的数据列表
print( [ele for ele in range(3,94,10)])
#?b. 利用列表推导式将已知列表中的整数提取出来
#?[17, 98, 34, 21]
list_two = [True, 17, "hello", "bye", 98, 34, 21]
print([ele for ele in list_two if type(ele) == int])
#?利用列表推导式存放指定列表中字符串的长度
#? --- [4, 4, 7, 3]
list_three=["good", "nice", "see you", "bye"]
print([len(ele) for ele in list_three])
#? d.生成一个列表,其中的元素为'0-1','1-2','2-3','3-4','4-5'
print([str(i)+"-"+str(i+1) for i in range(0,5)])
print([f"{i}_{i+1}" for i in range(0,5)])
5. dict_list = [{“科目”:“政治”, “成绩”:98}, {“科目”:“语文”, “成绩”:77}, {“科目”:“数学”, “成绩”:99}, {“科目”:“历史”, “成绩”:65}],
# 去除列表中成绩小于70的字典 【列表推导式完成】
dict_list = [
{"科目":"政治", "成绩":98},
{"科目":"语文", "成绩":77},
{"科目":"数学", "成绩":99},
{"科目":"历史", "成绩":65}]
dict_list.remove([ele for ele in dict_list[::] if ele["成绩"]<70][0])
print(dict_list)
6.用三个列表表示三门学科的选课学生姓名(一个学生可以同时选多门课)
subject_list = {
# a,b,c...分别代表学生姓名,1,2,3代表课程
'a': ['1', '2', '3'],
'b': ['1', '3'],
'c': ['1', '2'],
'd': ['3'],
'e': ['2', '3'],
'f': ['2', '3']
}
#?a. 求选课学生总共有多少人
print(f"选课学生共有:{len(subject_list)}人")
#?b. 求只选了第一个学科的人的数量和对应的名字
#?c. 求只选了一门学科的学生的数量和对应的名字
#?d. 求只选了两门学科的学生的数量和对应的名字
#?e. 求选了三门学生的学生的数量和对应的名字
count_one = 0
count_only = 0
count_two = 0
count_only_three = 0
for k,v in subject_list.items():
if "1" in v:
count_one +=1
print(f"选择第一个学科的名字是 :{k}")
print(f"选课第一个学科的人的数量: {count_one}")
print("-------------------------'")
for k,v in subject_list.items():
if len(v) == 1:
count_only +=1
print(f"只选了一门学科的学生名字是 :{k}")
print(f"只选了一门学科的学生数量: {count_only}")
print("-------------------------'")
for k,v in subject_list.items():
if len(v) == 2:
count_two +=1
print(f"只选了两门学科的学生名字是 :{k}")
print(f"只选了两门学科的学生的数量: {count_two}")
print("-------------------------'")
for k,v in subject_list.items():
if len(v) == 3:
count_only_three +=1
print(f"三门学生的学生名字是 :{k}")
print(f"三门学生的学生的数量数量: {count_only_three}")
print("-------------------------'")
以上的练习题,从简到难,都是我学习过程中的一些练习题,提供给正在学习Python的小伙伴,想要做的话,一定要自己手敲一遍,我写的答案是正确的,但是每个人的思维是不一样的,欢迎一起探讨。
更多推荐
所有评论(0)