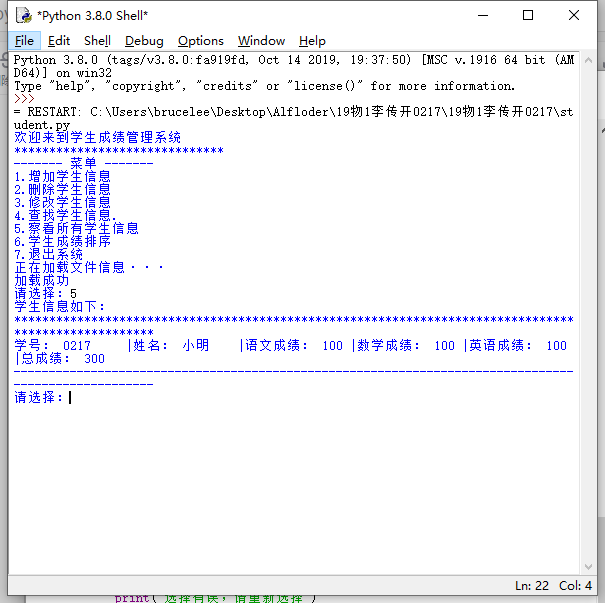
python学生成绩管理系统
python学生成绩管理系统描述程序源代码描述学生成绩管理系统可以实现学生基本信息的管理:主要实现以下功能:1.输入并存储 信息到文件(包含学生的学号、姓名和分数);2.输出学生信息并显示在屏幕上;3.具有查询功能 判断该学生是否存在,若存在,能实现对该学生的信息进行修改及删除等功能;4.实现按 学生成绩高低的排序功能;5.实现查找学生信息功能。程序源代码#import pickleimport
·
描述
学生成绩管理系统可以实现学生基本信息的管理:主要实现以下功能:
1.输入并存储 信息到文件(包含学生的学号、姓名和分数);
2.输出学生信息并显示在屏幕上;
3.具有查询功能 判断该学生是否存在,若存在,能实现对该学生的信息进行修改及删除等功能;
4.实现按 学生成绩高低的排序功能;
5.实现查找学生信息功能。
程序源代码
#import pickle
import os
stulist=[]#新建学生列表存储学生对象
class stu:
def __init__(self):
self.name=""
self.id=""
self.score1=0 #语文
self.score2=0 #数学
self.score3=0 #英语
self.sum=0 #总分
def sumscore(self):
self.sum=self.score1+self.score2+self.score3
return self.sum
def output(self,file_object):
file_object.write(self.name)
file_object.write(" ")
file_object.write(self.id)
file_object.write(" ")
file_object.write(str(self.score1))
file_object.write(" ")
file_object.write(str(self.score2))
file_object.write(" ")
file_object.write(str(self.score3))
file_object.write(" ")
file_object.write(str(self.sum))
file_object.write("\n")
#添加学生信息
def addstu():
student=stu()
student.name=input("请输入学生姓名:")
student.id=input("请输入学生id:")
if(cfindstu(student.id)!=-1):
print("该学生已存在,添加失败")
return False
student.score1=int(input("请输入学生语文成绩:"))
student.score2=int(input("请输入学生数学成绩:"))
student.score3=int(input("请输入学生英语成绩:"))
stulist.append(student)
print("添加成功!")
return True
#查找学生信息
def findstu():
idin=input("请输入学生学号:")
for i in range(0,len(stulist)):
if(idin==stulist[i].id):
print("该学生信息如下:")
print("学号:",stulist[i].id,end="\t|")
print("姓名:",stulist[i].name,end="\t|")
print("语文成绩:",stulist[i].score1,end="\t|")
print("数学成绩:",stulist[i].score2,end="\t|")
print("英语成绩:",stulist[i].score3,end="\t|")
print("总成绩:",stulist[i].sumscore())
return True
print("没有该学生信息")
return False
#查重 已存在:返回列表中坐标;不存在:返回-1
def cfindstu(idin):
for i in range(0,len(stulist)):
if(idin==stulist[i].id):
return i
return -1
#查看所有学生信息
def checkstu():
print("学生信息如下:")
print("*"*100)#换行
if len(stulist)==0:
print("当前无学生信息")
for i in range(0,len(stulist)):
print("学号:",stulist[i].id,end="\t|")
print("姓名:",stulist[i].name,end="\t|")
print("语文成绩:",stulist[i].score1,end="\t|")
print("数学成绩:",stulist[i].score2,end="\t|")
print("英语成绩:",stulist[i].score3,end="\t|")
print("总成绩:",stulist[i].sumscore())
print("-"*100)#换行
#删除学生信息
def delstu():
idin=input("请输入你要删除学生的学号:")
#利用查重函数返回删除学生在列表中坐标
result=cfindstu(idin)
if result==-1:
print("该学生不存在")
else:
for i in range(result,len(stulist)-1):
stulist[i]=stulist[i+1]
del stulist[len(stulist)-1]
print("删除成功")
#修改学生信息
def changestu():
idin=input("请输入你要修改学生的学号:")
result=cfindstu(idin)
if result==-1:
print("该学生不存在")
else:
id=input("请重新输入学生id:")
if(cfindstu(id)!=-1):
print("该id已存在,修改失败")
return False
stulist[result].id=id
stulist[result].name=input("请重新输入学生姓名:")
stulist[result].score1=int(input("请重新输入学生语文成绩:"))
stulist[result].score2=int(input("请重新输入学生数学成绩:"))
stulist[result].score3=int(input("请重新输入学生英语成绩:"))
print("修改成功,按“5”查看")
#按学生成绩排序,采用插入法
def sortstu():
for i in range(0,len(stulist)-1):
for j in range(i+1,0,-1):
if stulist[j].sum > stulist[j-1].sum:
tmp=stu()
tmp=stulist[j-1]
stulist[j-1]=stulist[j]
stulist[j]=tmp
print("排序成功!")
checkstu()
#将学生信息保存到文件
def writeinfo():
if os.path.exists('students.txt'):
os.remove('students.txt')
i=0
while i<len(stulist):
file_object=open("students.txt","a")
stulist[i].output(file_object)
file_object.close()
i+=1
#将学生信息读取到内存
def readinfo():
if os.path.exists('students.txt'):
file_object=open('students.txt','r')
for line in file_object:
stu1=stu()
line=line.strip("\n")
s=line.split(" ")
stu1.name=s[0]
stu1.id=s[1]
stu1.score1=int(s[2])
stu1.score2=int(s[3])
stu1.score3=int(s[4])
stu.sum=int(s[5])
stulist.append(stu1)
file_object.close()
print("加载成功")
else:
print("没有学生信息,请添加")
def main():
print("欢迎来到学生成绩管理系统")
print("*"*30)
print("-"*7,"菜单","-"*7)
print("1.增加学生信息")
print("2.删除学生信息")
print("3.修改学生信息")
print("4.查找学生信息.")
print("5.察看所有学生信息")
print("6.学生成绩排序")
print("7.退出系统")
print("正在加载文件信息···")
readinfo()#读取文件信息
while True:
writeinfo()#保存到文件
a=input("请选择:")
if(a=="1"):
addstu()
elif a=="2":
delstu()
elif a=="3":
changestu()
elif(a=="4"):
findstu()
elif(a=="5"):
checkstu()
elif a=="6":
sortstu()
elif a=="7":
print("已退出!")
print("文件信息已更新!")
break;
else:
print("选择有误,请重新选择")
main()
更多推荐
所有评论(0)