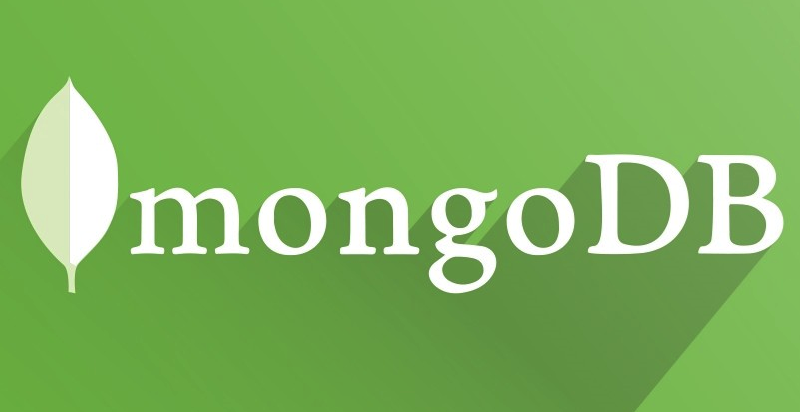
Java语言连接MongoDB常用的方法
Java连接操作mongoDB
·
MongoDB是我们常用的非关系型数据库之一,今天我们了解一下如何使用Java去连接使用MongoDB。
1.导入MongoDB驱动包
2.获取Mongo连接对象
MongoClient mc = new MongoClient("localhost",27017);
3.查看库、集合(表)
//从链接对象中拿到库对象
MongoDatabase database = mc.getDatabase("库名");
//从库对象中拿到某个集合(表)
MongoCollection<Document> collection = database.getCollection("表名");
4.查看集合中的数据
//调用find方法,拿到表中的数据,是一个二进制格式的文档
FindIterable<Document> find = collection.find();
//将拿到的文档进行迭代器迭代,拿到迭代器对象
MongoCursor<Document> iterator = find.iterator();
//hasNext进行判断是否有下一条
while(iterator.hasNext()) {
Document next = iterator.next();
System.out.println(next);
}
5.关闭连接
mc.close();
在Java中进行MongoDB的增删改查
首先我们需要拿到库对象和集合对象,接下来我们的库对象以myschool为例,集合对象以student为例
1.增加数据
(1)增加一条数据
public static void main(String[] args) {
//创建实体类对象给其赋值
Student s = new Student();
s.setSid(200);
s.setSname("马三娘");
s.setBirthday(new Date());
s.setSex("女");
s.setClassid(3);
//拿到链接对象
MongoClient mc = new MongoClient("localhost", 27017);
//从链接对象中拿到库对象
MongoDatabase database = mc.getDatabase("myschool");
//从库对象中拿到某个集合(表)
MongoCollection<Document> collection = database.getCollection("student");
//拿到document对象,将实体类对象传入document对象
Document doc = new Document();
doc.put("sid", s.getSid());
doc.put("sname", s.getSname());
doc.put("birthday", s.getBirthday());
doc.put("sex", s.getSex());
doc.put("classid", s.getClassid());
//将document对象新增到表中, 新增一条数据
collection.insertOne(doc);
//释放资源
mc.close();
}
(2)增加多条数据
//存入数据
List<Document> dlist=new ArrayList<Document>();
for(int i=0; i<3; i++){
Student s = new Student();
s.setSid(Integer.toString(i+1));
s.setSname("虹猫");
s.setBirthday(new Date());
s.setSsex("男");
s.setClassid(1);
//将数据转换为json格式
Gson gson = new GsonBuilder().setDateFormat("yyyy-MM-dd").create();
String json = gson.toJson(s);
dlist.add(Document.parse(json));
}
//获取集合对象
MongoCollection<Document> collection = db.getCollection("student");
//添加多条数据
collection.insertMany(dlist);
2.删除数据
(1)删除一条数据
// Filters 通过提供的条件方法得到 Bson 对象
Bson bson = Filters.eq("sid", 1);
//处理mongo
MongoClient mc = new MongoClient("localhost",27017);
MongoDatabase database = mc.getDatabase("myschool");
MongoCollection<Document> collection = database.getCollection("student");
DeleteResult deleteOne = collection.deleteOne(bson);
(2)删除多条数据
//获取集合对象
MongoCollection<Document> collection = db.getCollection("student");
Student s = new Student();
s.setSname("虹猫");
Gson gson = new GsonBuilder().setDateFormat("yyyy-MM-dd").create();
Bson bson = Document.parse(gson.toJson(s));
DeleteResult deleteMany = collection.deleteMany(bson);
3.修改数据
(1)修改一条数据
//条件对象
Bson eq = Filters.eq("sname", "王五");
//要修改的数据
Document doc1 = new Document();
doc1.put("sex", "男");
Document doc2 = new Document();
doc2.put("$set", doc1);
//调用修改方法
UpdateResult updateOne = collection.updateOne(eq, doc2);
(2)修改多条数据
//条件对象
Bson and = Filters.and(Filters.gte("age", 21),Filters.lte("age", 50));
//需要修改的数据
Document doc1 = new Document();
doc1.put("sex", "男");
UpdateResult updateMany = collection.updateMany(and, new Document("$set",doc1));
4.查找数据
(1)全查
MongoCollection<Document> collection = db.getCollection("student");
FindIterable<Document> findAll = collection.find();
MongoCursor<Document> iterator = findAll.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
(2)条件查询(需要得到一个Bson对象)
MongoCollection<Document> collection = db.getCollection("student");
//一个条件对象
Bson eq = Filters.eq("sname","马三娘");
FindIterable<Document> findOne = collection.find(eq);
MongoCursor<Document> iterator = findOne.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
(3)分页查询(需要用到 skip() 、limit() 方法)
MongoCollection<Document> collection = db.getCollection("student");
//分页查询
FindIterable<Document> find = collection.find().skip(3).limit(3);
MongoCursor<Document> iterator = find.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
(4)模糊查询(需要使用正则表达式)
MongoCollection<Document> collection = db.getCollection("student");
//使用正则表达式进行模糊查找
Bson eq = Filters.regex("sname","三");
FindIterable<Document> find = collection.find(eq);
MongoCursor<Document> iterator = find.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
(5)排序(1 代表升序 -1 代表降序 需要用到 sort() 方法)
MongoCollection<Document> collection = db.getCollection("student");
//通过sid进行排序 1升序 -1降序
Bson bson = new Document("sid",1);
FindIterable<Document> find = collection.find().sort(bson);
MongoCursor<Document> iterator = find.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
更多推荐
所有评论(0)