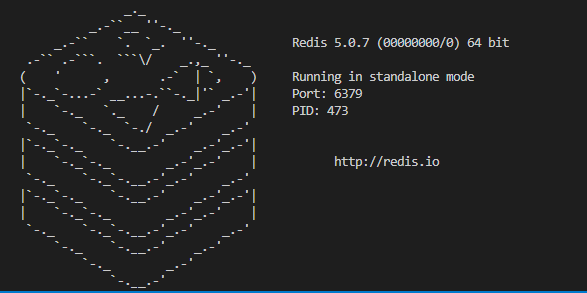
用C++连接redis数据库
C++连接redis
·
环境:ubuntu 20.04
1、先确保开启redis-server
sudo apt-get install redis #安装reids
redis-server #启动redis服务端
redis-cli #本地客户端测试连接
2、安装hiredis
git clone https://github.com/redis/hiredis.git
cd hiredis
make
make install
3、redis测试代码
// redis.h
#include <iostream>
#include <hiredis/hiredis.h>
class Redis {
public:
Redis() = default;
~Redis() {
_connect = nullptr;
_reply = nullptr;
}
bool connect(std::string host, int port) {
_connect = redisConnect(host.c_str(), port);
if(_connect != nullptr && _connect->err) {
std::cout << "connect error: " << _connect->errstr << std::endl;
return false;
}
return true;
}
void set(std::string key, std::string value) {
redisCommand(_connect, "SET %s %s", key.c_str(), value.c_str());
}
std::string get(std::string key) {
_reply = static_cast<redisReply*>(redisCommand(_connect, "GET %s", key.c_str()));
std::string str = _reply->str;
freeReplyObject(_reply);
return str;
}
private:
redisContext* _connect;
redisReply* _reply;
};
// test.cpp
#include "redis.h"
int main() {
Redis *r = new Redis();
if(!r->connect("127.0.0.1", 6379)) {
printf("connect error!\n");
return 0;
}
r->set("name", "wuyi");
printf("Get the name is %s\n", r->get("name").c_str());
delete r;
return 0;
}
编译并运行
g++ test.cpp -lhiredis -o redis
./redis
参考
C++操作Redis数据库_ACHelloWorld的博客-CSDN博客
更多推荐
所有评论(0)