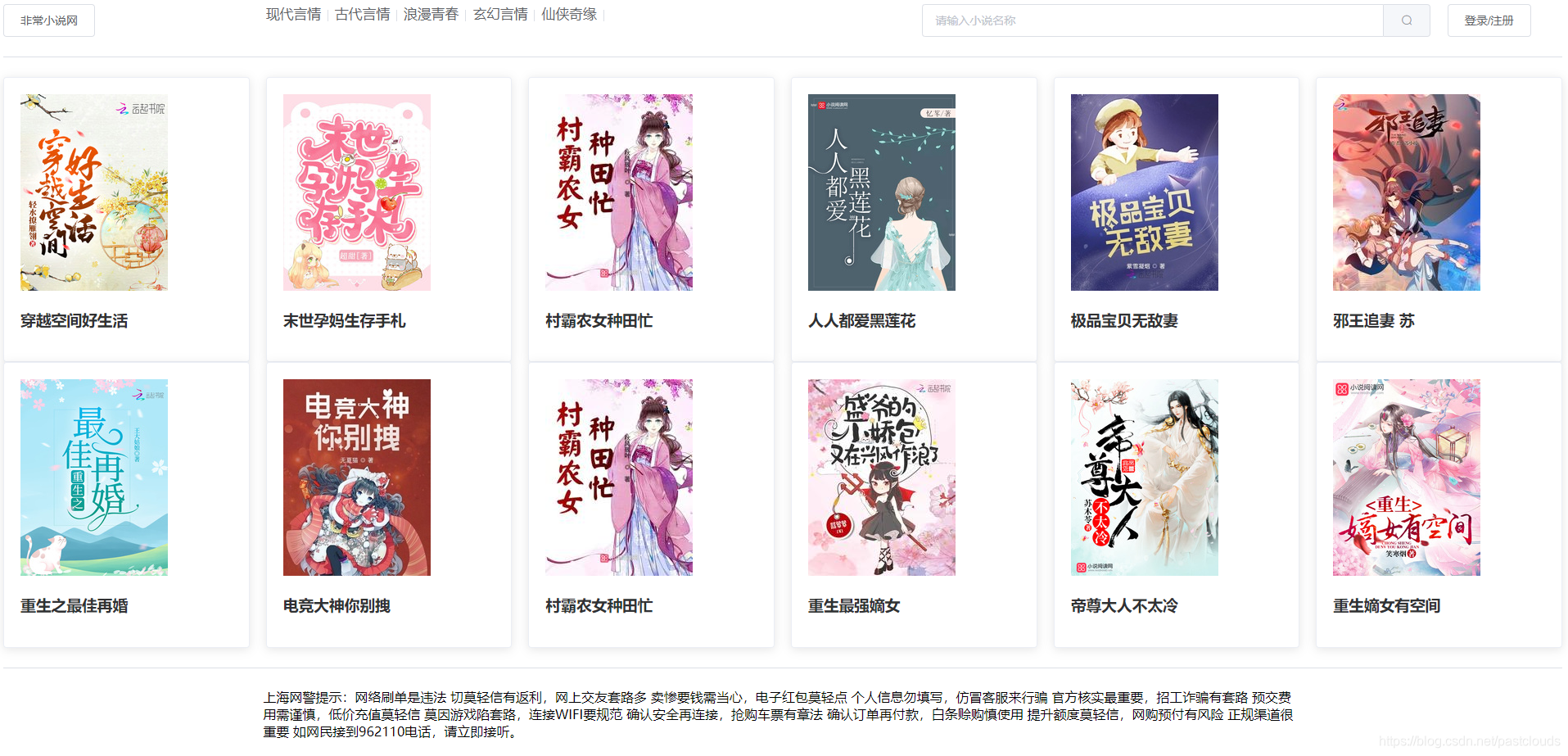
Java项目:在线小说阅读系统(前后端分离+java+vue+Springboot+ssm+mysql+maven+redis)
一、项目简述本系统功能包括: 普通用户端登录注册,小说的分类,日榜,月榜,年榜, 小说的阅读,分章节,小说的评论,收藏,推荐等等,以 及后台小说的维护,上架,编辑等等。二、项目运行环境配置: Jdk1.8 + Tomcat8.5 + Mysql + HBuilderX (Webstorm也 行)+ Eclispe (IntelliJ IDEA,Eclispe,MyEclispe,Sts都支 持)。
·
源码获取:博客首页 "资源" 里下载!
一、项目简述
本系统功能包括: 普通用户端登录注册,小说的分类,日榜,月榜,年榜, 小说的阅读,分章节,小说的评论,收藏,推荐等等,以 及后台小说的维护,上架,编辑等等。
二、项目运行
环境配置: Jdk1.8 + Tomcat8.5 + Mysql + HBuilderX (Webstorm也 行)+ Eclispe (IntelliJ IDEA,Eclispe,MyEclispe,Sts都支 持)。
项目技术: Springboot + Maven + Mybatis + Vue + Redis, B/S 模式+ Maven等等
商品分类控制器:
@RestController
@RequestMapping("/api/category")
@Api(tags = "共同前缀:/api/category", description = "CategoryController")
@Slf4j
public class CategoryController {
@Autowired
CategoryService categoryService;
@PostMapping
@ApiOperation("新增Category")
@PreAuthorize("hasAnyAuthority('ROLE_ADMIN')")
public ResponseObject post(@RequestBody Category category) {
log.info("新增Category");
if (category.getId() != null) {
throw new ControllerException("id必须为null");
} else if (category.getRank() == null) {
throw new ControllerException("rank不可为null");
} else if (category.getName() == null || category.getName().equals("")) {
throw new ControllerException("name不可为null,也不可为空字符串");
} else if (category.getParent_id() == null) {
throw new ControllerException("parent_id不可为null");
} else if (categoryService.selectByParent_idName(category.getParent_id(), category.getName()) != null) {
throw new ControllerException("name不可重复");
} else {
return new ResponseObject("200", "操作成功", categoryService.insert(category));
}
}
@PutMapping("/{id:[0-9]+}")
@ApiOperation("修改Category")
@PreAuthorize("hasAnyAuthority('ROLE_ADMIN')")
public ResponseObject putById(@PathVariable Integer id, @RequestBody HashMap<String, String> data) {
log.info("修改Category");
Category category = categoryService.selectById(id);
if (category == null) {
throw new ControllerException("使用该id的Category不存在");
} else if (categoryService.selectByParent_idName(category.getParent_id(), data.get("name")) != null) {
throw new ControllerException("同一个分类下的name不可重复");
} else {
category.setName(data.get("name"));
return new ResponseObject("200", "操作成功", categoryService.update(category));
}
}
@GetMapping
@ApiOperation("查询Category")
public ResponseObject getByParent_id(Integer rank, Integer parent_id) {
log.info("查询Category");
if (rank != null && parent_id != null) {
return new ResponseObject("200", "操作成功", categoryService.selectByRankParent_id(rank, parent_id));
} else if (rank != null && parent_id == null) {
return new ResponseObject("200", "操作成功", categoryService.selectByRank(rank));
} else if (rank == null && parent_id != null) {
return new ResponseObject("200", "操作成功", categoryService.selectByParent_id(parent_id));
} else {
throw new ControllerException("rank或者parent_id至少要有一个不为null");
}
}
@GetMapping("/{id:[0-9]+}")
@ApiOperation("查询Category")
public ResponseObject getById(@PathVariable Integer id) {
log.info("查询Category");
if (id != null) {
return new ResponseObject("200", "操作成功", categoryService.selectById(id));
} else {
throw new ControllerException("id不可为null");
}
}
}
用户信息控制器:
@RestController
@RequestMapping("/api/user")
@Api(tags = "共同前缀:/api/user", description = "UserController")
@Slf4j
public class UserController {
@Autowired
UserService userService;
@PostMapping
@ApiOperation("新增User")
public ResponseObject post(String username, String password) {
log.info("新增User");
if (username == null || username.equals("")) {
throw new ControllerException("username不可为null,也不可为空字符串");
} else if (password == null || password.equals("")) {
throw new ControllerException("password不可为null,也不可为空字符串");
} else if (userService.selectByUsername(username) != null) {
throw new ControllerException("该username已被使用");
} else {
User user = new User();
user.setUsername(username);
user.setPassword(password);
user.setRole("VIP1");
user.setNickname(new Date().getTime() + "");
user.setImage("default_user_image.png");
user.setEmail("该用户没有填写邮箱");
user.setPhone("该用户没有填写手机号码");
user.setProfile("该用户没有填写个人简介");
return new ResponseObject("200", "操作成功", userService.insert(user));
}
}
@GetMapping("/{id:[0-9]+}")
@ApiOperation("查询User")
public ResponseObject getById(@PathVariable Integer id) {
log.info("查询User");
return new ResponseObject("200", "操作成功", userService.selectById(id));
}
@PutMapping
@ApiOperation("修改User")
@PreAuthorize("isAuthenticated()")
public ResponseObject put(User user) {
log.info("修改User");
if (user.getNickname() == null || user.getNickname().equals("")) {
throw new ControllerException("nickname不可为null,也不可为空字符串");
} else if (user.getProfile() == null || user.getProfile().equals("")) {
throw new ControllerException("profile不可为null,也不可为空字符串");
} else if (user.getPhone() == null || user.getPhone().equals("")) {
throw new ControllerException("phone不可为null,也不可为空字符串");
} else if (user.getEmail() == null || user.getEmail().equals("")) {
throw new ControllerException("email不可为null,也不可为空字符串");
} else {
User user2 = userService
.selectByUsername((String) SecurityContextHolder.getContext().getAuthentication().getPrincipal());
user2.setNickname(user.getNickname());
user2.setProfile(user.getProfile());
user2.setPhone(user.getPhone());
user2.setEmail(user.getEmail());
return new ResponseObject("200", "操作成功", userService.update(user2));
}
}
}
收藏控制器:
@RestController
@RequestMapping("/api/collection")
@Api(tags = "共同前缀:/api/collection", description = "CollectionController")
@Slf4j
public class CollectionController {
@Autowired
CollectionService collectionService;
@Autowired
UserService userService;
@Autowired
NovelService novelService;
@PostMapping
@ApiOperation("新增Collection")
@PreAuthorize("isAuthenticated()")
public ResponseObject post(Collection collection) {
log.info("新增Collection");
if (collection.getId() != null) {
throw new ControllerException("id必须为null");
} else if (collection.getNovel_id() == null) {
throw new ControllerException("novel_id不可为null");
} else {
collection.setUser_id(userService
.selectByUsername((String) SecurityContextHolder.getContext().getAuthentication().getPrincipal())
.getId());
if (collectionService.selectByUser_idNovel_id(collection.getUser_id(), collection.getNovel_id()) != null) {
throw new ControllerException("该用户已经收藏过该小说了,不可重复收藏");
} else {
return new ResponseObject("200", "操作成功", collectionService.insert(collection));
}
}
}
@GetMapping
@ApiOperation("查询Collection")
public ResponseObject get(Integer novel_id, Integer user_id) {
log.info("查询Collection");
if (novel_id != null && user_id != null) {
return new ResponseObject("200", "操作成功", collectionService.selectByUser_idNovel_id(user_id, novel_id));
} else if (novel_id != null && user_id == null) {
return new ResponseObject("200", "操作成功", collectionService.selectByNovel_id(novel_id));
} else if (novel_id == null && user_id != null) {
return new ResponseObject("200", "操作成功", collectionService.selectByUser_id(user_id));
} else {
throw new ControllerException("novel_id与user_id不可同时为null");
}
}
@GetMapping("/novel")
@ApiOperation("查询Collection的Novel")
public ResponseObject getNovel(Integer user_id) {
log.info("查询Collection的Novel");
if (user_id == null) {
throw new ControllerException("user_id不可为null");
} else {
return new ResponseObject("200", "操作成功", novelService.selectByUser_idOfCollection(user_id));
}
}
@DeleteMapping
@ApiOperation("删除Collection")
@PreAuthorize("isAuthenticated()")
public ResponseObject delete(Integer novel_id, Integer user_id) {
log.info("删除Collection");
if (novel_id == null) {
throw new ControllerException("novel_id不可为null");
} else if (user_id == null) {
throw new ControllerException("user_id不可为null");
} else {
Collection collection = collectionService.selectByUser_idNovel_id(user_id, novel_id);
if (collection == null) {
throw new ControllerException("该用户还未收藏该小说,无法取消收藏");
} else {
User user = userService.selectByUsername(
(String) SecurityContextHolder.getContext().getAuthentication().getPrincipal());
if (user.getId() == user_id || user.getRole().equals("ADMIN")) {
collectionService.deleteById(collection.getId());
return new ResponseObject("200", "操作成功", null);
} else {
throw new ControllerException("该用户无权限取消收藏");
}
}
}
}
@GetMapping("/count")
@ApiOperation("查询Collection")
public ResponseObject getCount(Integer novel_id) {
log.info("查询Collection");
if (novel_id == null) {
throw new ControllerException("novel_id不可为null");
} else {
return new ResponseObject("200", "操作成功", collectionService.selectByNovel_id(novel_id).size());
}
}
}
源码获取:博客首页 "资源" 里下载!
更多推荐
所有评论(0)