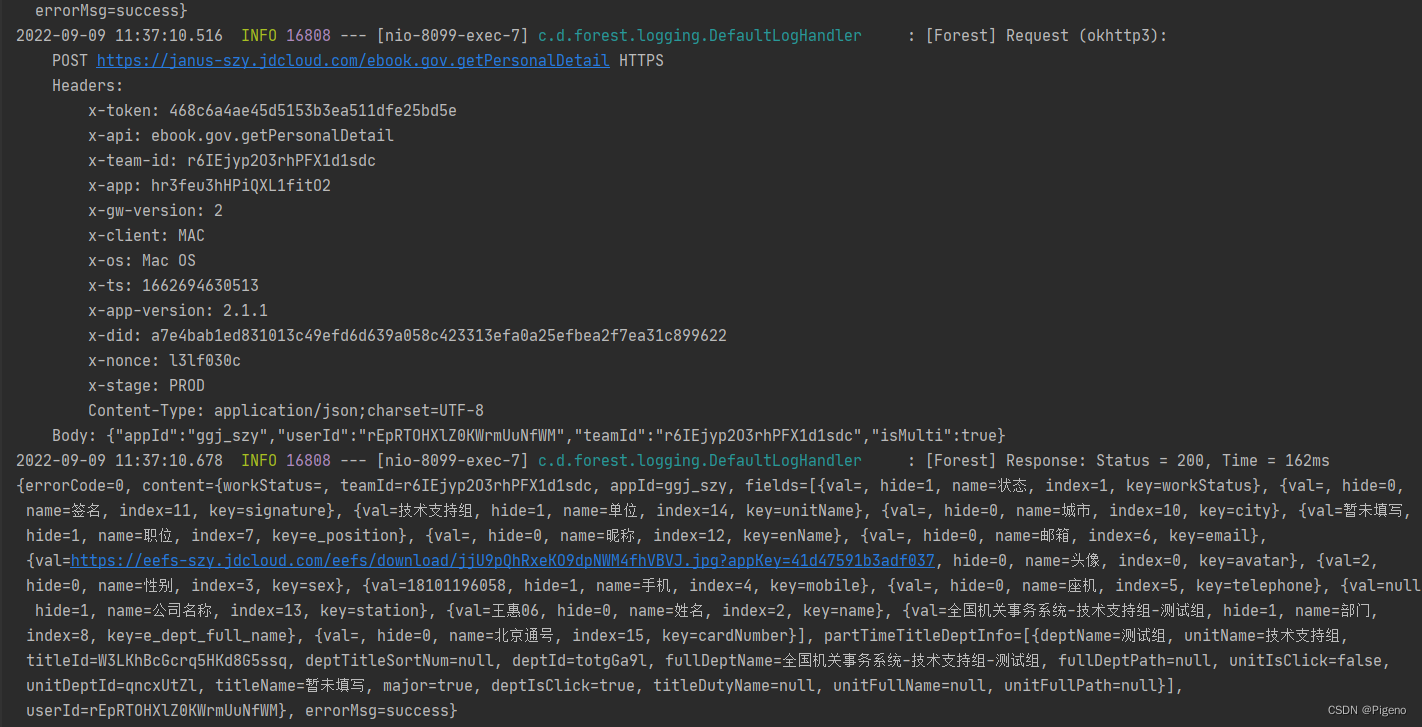
springboot + yml + redis + HttpClient 实现自动化测试
springboot + yml + redis + HttpClient 实现自动化测试
·
springboot + yml + redis + HttpClient 实现自动化测试
原创,请勿转载!!!!!
要是实现了,帮忙点个赞!!!!!
目录
1、使用技术;
2、jar包依赖;
3、准备;
4、实现效果。
1、使用技术;
1、springbot 框架;
2、forest-spring-boot-starter http请求jar包;
3、redis;
4、Yml文件;
2、jar包依赖;
父级依赖springboot
<dependencies>
<dependency>
<groupId>com.dtflys.forest</groupId>
<artifactId>forest-spring-boot-starter</artifactId>
<version>1.5.13</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.yaml</groupId>
<artifactId>snakeyaml</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
</dependency>
</dependencies>
3、准备;
3.1 HttpClient
import com.dtflys.forest.annotation.*;
/**
* @author yinxing
* @date 2022-08-23
*/
public interface ClientHttp {
/**
* Get请求
* @param url 访问的url地址
* @param header 请求头信息
*/
@Get(url = "{0}")
public Object sendGet(String url, @Header Object header);
/**
* Post请求
* @param url 请求url地址
* @param header 请求头信息
* @param param 请求参数,JSON转换
*/
@Post(url = "{0}")
public Object sendPost(String url,@Header Object header,@JSONBody Object param);
}
2、Yml文件固定格式
#Get请求接口示列
test:
type: Get
url: http://localhost:8003/hello
header: {
yx-token: keyi,
abc: aaa
}
request: {
source: "shop"
}
response: {
code: 200,
message: success
}
#Post请求接口示列
testPost:
type: Post
url: http://localhost:8003/testPost
header: {
yx-token: keyi,
abc: aaa
}
request: {
abc: "av"
}
response: {
code: 200,
message: success
}
3.3HttpService
/**
* @author yinxing
* @date 2022-08-24
*/
public interface HttpService {
/**
* case请求
* @param fileName
* @param methodName
* @return
* @throws FileNotFoundException
*/
public String caseHttp(String fileName,String methodName) throws FileNotFoundException;
/**
* 登录
* @return
* @throws FileNotFoundException
*/
String login() throws FileNotFoundException;
}
3.4HttpServiceImpl
import com.yx.auto.contanct.Constant;
import com.yx.auto.http.ClientHttp;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
import org.yaml.snakeyaml.Yaml;
import javax.annotation.Resource;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* @author yinxing
* @date 2022-08-24
*/
@Service
public class HttpServiceImpl implements HttpService{
@Resource
private ClientHttp clientHttp;
@Resource
private RedisTemplate<String, String> redisTemplate;
@Override
public String caseHttp(String fileName,String methodName) throws FileNotFoundException {
Yaml yaml = new Yaml();
FileReader fileReader = new FileReader(this.getClass().getClassLoader().getResource(fileName).getPath());
BufferedReader buffer = new BufferedReader(fileReader);
Map<String, Object> map = yaml.load(buffer);
Map<String, Object> method;
method = (Map<String, Object>) map.get(methodName);
if (method.get(Constant.type).equals(Constant.get)) {
Object o = clientHttp.sendGet(method.get(Constant.header).toString(), method.get(Constant.header));
System.out.println(o.toString());
} else {
Map<String, Object> header = (Map<String, Object>) method.get(Constant.header);
for (Map.Entry<String, Object> headerOne : header.entrySet()) {
if (headerOne.getKey().contains("x-ts")) {
header.put("x-ts", System.currentTimeMillis());
}
if (headerOne.getValue().toString().contains(Constant.redis)){
String split = headerOne.getValue().toString().
substring(headerOne.getValue().toString().lastIndexOf('.')+1);
header.put(headerOne.getKey(),redisTemplate.opsForValue().get(split));
}
}
Map<String, Object> requests = (Map<String, Object>) method.get(Constant.request);
for (Map.Entry<String, Object> request : requests.entrySet()) {
if (request.getValue().toString().contains(Constant.redis)){
String split = request.getValue().toString().
substring(request.getValue().toString().lastIndexOf('.')+1);
requests.put(request.getKey(),redisTemplate.opsForValue().get(split));
}
}
Object o = clientHttp.sendPost(method.get(Constant.url).toString(), header, requests);
System.out.println(o.toString());
}
return "success";
}
@Override
public String login() throws FileNotFoundException {
Yaml yaml = new Yaml();
FileReader fileReader = new FileReader(this.getClass().getClassLoader().getResource("login.yml").getPath());
BufferedReader buffer = new BufferedReader(fileReader);
Map<String, Object> map = yaml.load(buffer);
Map<String, Object> method = (Map<String, Object>) map.get("login");
if (method.get("type").equals("Get")) {
Object o = clientHttp.sendGet(method.get("url").toString(), method.get("header"));
System.out.println(o.toString());
} else {
Map<String, Object> header = (Map<String, Object>) method.get("header");
if (header.get("x-ts") != null) {
header.put("x-ts", System.currentTimeMillis());
}
if (header.get("accessToken") != null) {
Object accessToken = redisTemplate.opsForValue().get("accessToken");
if (accessToken == null) {
}
header.put("accessToken", redisTemplate.opsForValue().get("accessToken"));
}
Map<String, Object> request = (Map<String, Object>) method.get("request");
Object o = clientHttp.sendPost(method.get("url").toString(), header, request);
System.out.println(o.toString());
Map<String, Object> result = (Map<String, Object>) o;
Map<String, Object> content = (Map<String, Object>) result.get("content");
redisTemplate.opsForValue().set("accessToken", content.get("accessToken").toString(), Long.parseLong(content.get("accessTokenExpireIn").toString()), TimeUnit.SECONDS);
redisTemplate.opsForValue().set("userId",content.get("userId").toString());
String accessToken = redisTemplate.opsForValue().get("accessToken");
System.out.println("登录凭证accessToken:" + accessToken);
}
return null;
}
}
4、实现效果。
4.1DemoController
import com.yx.auto.http.ClientHttp;
import com.yx.auto.service.HttpService;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import java.io.FileNotFoundException;
/**
* @author yinxing
* @date 2022-08-23
*/
@RestController
public class DemoController {
@Resource
private HttpService httpService;
/**
* szy
*
* @return
* @throws FileNotFoundException
*/
@RequestMapping("/szy")
public String login() throws FileNotFoundException {
httpService.login();
//调用接口,yml文件名,方法名
httpService.caseHttp("szy.yml", "getEbookDeptInfo");
httpService.caseHttp("demo.yml", "getPersonalDetailfromebook");
return "Success";
}
}
更多推荐
所有评论(0)