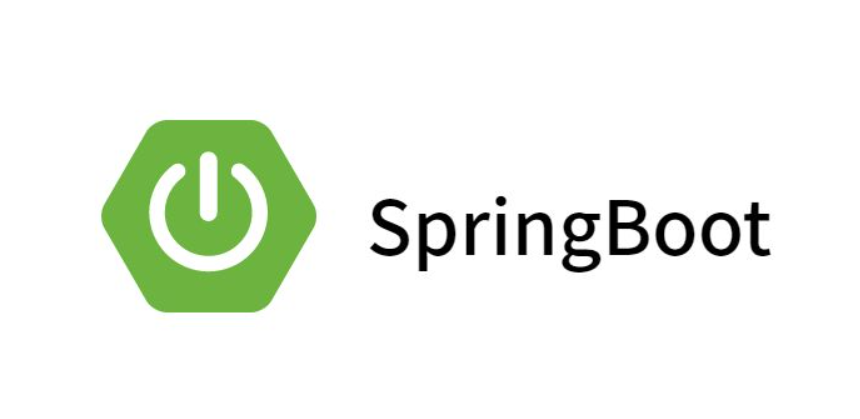
springboot基础(42):jetCache缓存方案
JetCache是对SpringCache进行了封装,在原有基础上实现了多级缓存、缓存统计、自动刷新、异步调用、数据报表等功能。JetCache设定了本地缓存与远程缓存的多级缓存方案。
·
文章目录
前言
JetCache是阿里推出的一套替代springcache的缓存方案。
JetCache是对SpringCache进行了封装,在原有基础上实现了多级缓存、缓存统计、自动刷新、异步调用、数据报表等功能。
JetCache设定了本地缓存与远程缓存的多级缓存方案
- 本地缓存
LinkedHashMap
Caffeine - 远程缓存
Redis
Tair
本地缓存和远程缓存可以任意组合。
jetcache官方源码: https://github.com/alibaba/jetcache
jetcache远程缓存方案
远程缓存(demo为redis)
本demo的springboot版本2.5.4
- 导入依赖
<!-- https://mvnrepository.com/artifact/com.alicp.jetcache/jetcache-starter-redis -->
<dependency>
<groupId>com.alicp.jetcache</groupId>
<artifactId>jetcache-starter-redis</artifactId>
<version>2.6.2</version>
</dependency>
- 配置
jetcache:
remote:
default:
type: redis
host: localhost
port: 6379
poolConfig:
maxTotal: 50
- 主类开启缓存注解
@SpringBootApplication
@EnableCreateCacheAnnotation //开启缓存
public class SpringbootMybatisplusApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootMybatisplusApplication.class, args);
}
}
- 使用
import com.alicp.jetcache.Cache;
import com.alicp.jetcache.anno.CreateCache;
import com.it2.springbootmybatisplus.pojo.SmsCode;
import com.it2.springbootmybatisplus.util.SmsUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/sms")
public class SmsCodeController {
@Autowired
private SmsUtils smsUtils;
@CreateCache(name="jetCache",expire = 3600)
private Cache<String,String> jetCache;
@GetMapping
public String getCode(String phone) {
String code= smsUtils.createCode(phone);
jetCache.put(phone,code);
return code;
}
@PostMapping
public boolean checkCode(SmsCode smsCode) {
String code = smsCode.getCode();
// String code2 = smsUtils.getCode(smsCode.getPhone());
String code2 = jetCache.get(smsCode.getPhone());
return code.equals(code2);
}
}
- 启动服务器测试
查看缓存可以看到,它的空间是default,而前缀是jetCache。
更换空间
配置area
@CreateCache(area = “sms”,name=“jetCache”,expire = 3600)
配置对应空间名的配置
运行后可以查看到redis显示的前缀为sms为空间
jetcache本地缓存方案
本地缓存(demo为linkedhashmap)
- 配置本地缓存
jetcache:
local:
default:
type: linkedhashmap
keyConvertor: fastjson #key的转换器
- 声明使用本地缓存
@CreateCache(name="jetCache",expire = 3600,cacheType= CacheType.LOCAL)
private Cache<String,String> jetCache;
- 启动服务器测试
默认的方案
默认的方案是REMOTE远程方案。可以只选择本地方案或者远程方案中的一种,也可以同时存在。
注解方式
注解方式demo(@Cached)
- 在主类配置缓存方法需要扫描的包
@SpringBootApplication
@EnableCreateCacheAnnotation //开启缓存
@EnableMethodCache(basePackages = {"com.it2"}) //配置缓存方法需要扫描的包
public class SpringbootMybatisplusApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootMybatisplusApplication.class, args);
}
}
- 注解方法
@Cached(name="book",key="#id",expire = 3600)
public Book queryById(Integer id) {
System.out.println("读取数据库:id="+id);
return bookMapper.selectById(id);
}
- 实现序列化接口Serializable,由于需要将Object缓存起来,所以需要实现序列化接口Serializable
@Data
public class Book implements Serializable {
private int id;
private String name;
private String type;
private String description;
}
- 修改配置文件
jetcache:
remote:
default:
type: redis
host: localhost
port: 6379
keyConvertor: fastjson #key的转换器
valueEncode: java
valueDecode: java
poolConfig:
maxTotal: 50
sms:
type: redis
host: localhost
port: 6379
poolConfig:
maxTotal: 50
local:
default:
type: linkedhashmap
keyConvertor: fastjson #key的转换器
- 启动服务器测试,发起请求,可以看到只有一次查询数据库操作
缓存更新(@CacheUpdate)
使用@CacheUpdate(name=“book_”,key=“#book.id”,value=“#book”)注解
@CacheUpdate(name="book_",key="#book.id",value="#book")
public boolean updateBook(Book book) {
return bookMapper.updateById(book)>0;
}
缓存删除(@CacheInvalidate)
使用@CacheInvalidate(name=“book_”,key=“#id”)注解
@CacheInvalidate(name="book_",key="#id")
public boolean deleteBook(Integer id) {
System.out.println("删除了数据:id="+id);
return true;
}
缓存刷新(@CacheRefresh)
refresh 设置缓存自动刷新的时间,间隔秒
@Cached(name="book_",key="#id",expire = 3600)
@CacheRefresh(refresh = 30)
public Book queryById(Integer id) {
System.out.println("读取数据库:id="+id);
return bookMapper.selectById(id);
}
缓存统计
配置缓存统计,并重启
jetcache:
statIntervalMinutes: 1 #缓存统计频率(分钟)
多次请求接口,在控制台查看统计缓存的命中信息
传送门
更多推荐
所有评论(0)