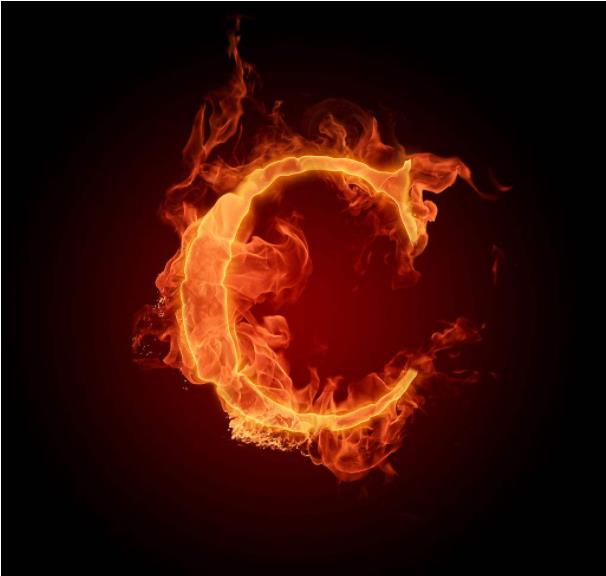
C语言---判断输入的日期是否合法
C语言,判断输入的日期是否合法,判断输入的日期范围是否合法
·
前提:本例中规定日期的格式为YYYY-MM-DD
1、判断单个日期是否合法
int check_date(int year, int month, int day)
{
int monthDays[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if ( year < 0 )
{
printf("the entered year[%d] is invalid\n", year);
return -1;
}
if ( month < 1 || month > 12 )
{
printf("The entered month[%d] is invalid\n", month);
return -1;
}
if ( month == 2 )
{
// 判断如果是闰年,则修改二月的monthDays[1]值为29
if ( (year % 400 == 0) || \
(year % 100 != 0 && year % 4 == 0)
)
{
monthDays[1] = 29;
}
}
if ( day < 1 || day > monthDays[month-1] )
{
printf("The entered day[%d] is invalid\n", day);
return -1;
}
return 0;
}
2、解析输入日期字符串YYYY-MM-DD,并判断日期范围是否合法
int is_valid_date(const char *startDate, const char *endDate)
{
int start_year = 0;
int start_month = 0;
int start_day = 0;
int end_year = 0;
int end_month = 0;
int end_day = 0;
if ( strlen(startDate) > 0 )
{
int count = 0;
printf("\n check start date... \n");
count = sscanf(startDate, "%4d-%2d-%2d", &start_year, &start_month, &start_day);
if ( count != 3 )
{
printf("Input parameters invalid.\n");
return -1;
}
if ( 0 != check_date(start_year, start_month, start_day) )
{
return -1;
}
printf("\n check start date OK!\n");
}
if ( strlen(endDate) > 0 )
{
int count = 0;
printf("\n check end date... \n");
count = sscanf(endDate, "%4d-%2d-%2d", &end_year, &end_month, &end_day);
if ( count != 3 )
{
printf("Input parameters invalid.\n");
return -1;
}
if ( 0 != check_date(end_year, end_month, end_day) )
{
return -1;
}
printf("\n check end date OK!\n");
}
printf("\n check date range...\n");
if ( strlen(startDate) > 0 && strlen(endDate) > 0 )
{
if ( (end_year < start_year) || \
(end_year == start_year && end_month < start_month) || \
(end_year == start_year && end_month == start_month && end_day < start_day)
)
{
printf("The end date is earlier than the start date!\n");
return -1;
}
}
printf("\n check date range OK!\n");
return 0;
}
3、测试代码
#include <stdio.h>
#include <string.h>
…… // is_valid_date()函数实现
int main(int argc, char **argv)
{
if ( argc != 3)
{
printf("Usage: %s <start-date> <end-date>\n", argv[0]);
return 0;
}
if ( 0 != is_valid_date(argv[1], argv[2]) )
{
printf("\n Input date is invalid!\n\n");
return -1;
}
printf("\n start-date[%s], end-date[%s] are valid!\n\n", argv[1], argv[2]);
return 0;
}
4、编译及测试
# gcc check_date.c -o check_date
#
#
# ./check_date 2022-2-3 2022-4-5
check start date...
check start date OK!
check end date...
check end date OK!
check date range...
check date range OK!
start-date[2022-2-3], end-date[2022-4-5] are valid!
#
#
#
更多推荐
所有评论(0)