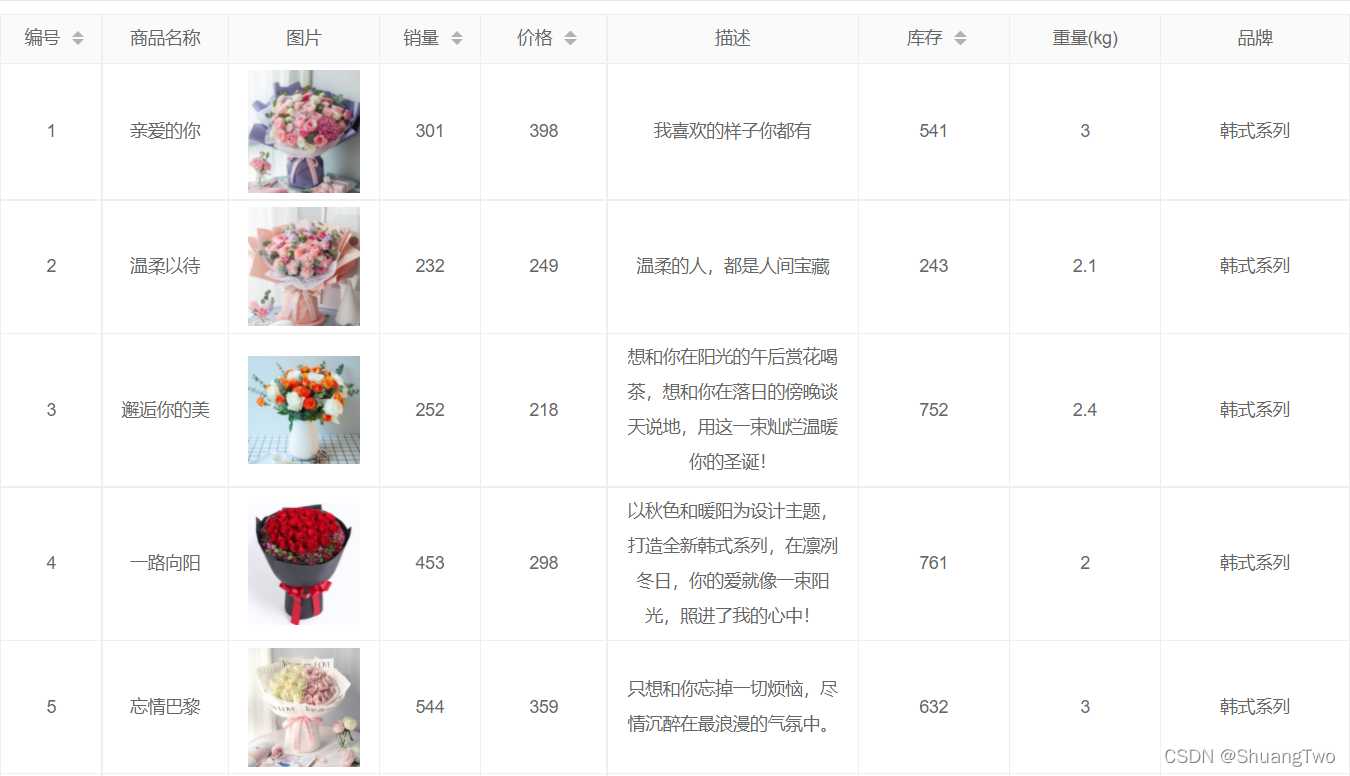
layUI框架表格中图片展示完整实例
整合layUI框架时,表格中的图片如何展示,以及展示图片遇到的问题。
·
一、建表,插入数据
CREATE TABLE `product` (
`id` bigint(0) NOT NULL AUTO_INCREMENT,
`name` varchar(64) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL COMMENT '商品名称',
`pic` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL COMMENT '图片',
`sale` int(0) NULL DEFAULT NULL COMMENT '销量',
`price` decimal(10, 2) NULL DEFAULT NULL COMMENT '价格',
`description` text CHARACTER SET utf8 COLLATE utf8_general_ci NULL COMMENT '商品描述',
`stock` int(0) NULL DEFAULT NULL COMMENT '库存',
`weight` decimal(10, 2) NULL DEFAULT NULL COMMENT '商品重量,默认为克',
`brand_name` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL COMMENT '品牌名称',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic;
二、SpringBoot查询所有商品业务代码
- pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.web</groupId>
<artifactId>layui-images</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>layui-images</name>
<description>layui-images</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
- application.yml
server:
port: 3000
spring:
datasource:
username: root
password: 123456
url: jdbc:mysql://localhost:3306/test?useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=Asia/Shanghai
thymeleaf:
cache: false
prefix:
classpath: /templates
suffix: .html
mybatis:
mapper-locations: classpath:mapper/*.xml
- 启动类添加注解扫描mapper接口:@MapperScan(“com.web.mapper”)
- Product实体类
package com.web.entity;
import lombok.Data;
@Data
public class Product {
private Integer id;
private String name;
private String pic;
private Integer sale;
private Double price;
private String description;
private Integer stock;
private Double weight;
private String brandName;
}
- 返回结果
package com.web.common;
import lombok.Data;
@Data
public class Result<T> {
/**
* 返回码,0表示成功,-1表示失败
*/
private Integer code;
/**
* 返回信息
*/
private String message;
/**
* 返回数据
*/
private T data;
/**
* 分页查询的总记录数
*/
private Long count;
/**
* 无参构造函数私有化
*/
private Result() {}
/**
* 全参构造函数私有化
*/
private Result(Integer code, String message, T data, Long count) {
this.code = code;
this.message = message;
this.data = data;
this.count = count;
}
public static Result<Object> success(Object data) {
return new Result(0, "success", data, null);
}
}
- ProductController控制层编写
package com.web.controller;
import com.web.common.Result;
import com.web.entity.Product;
import com.web.service.ProductService;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.annotation.Resource;
import java.util.List;
@Controller
public class ProductController {
@Resource
private ProductService productService;
@GetMapping("list")
public String toProductListUI() {
return "productList";
}
@GetMapping("/product")
@ResponseBody
public Result<Object> getProductList() {
List<Product> list = productService.getAllProduct();
return Result.success(list);
}
}
- service层—>service实现层—>mapper层
//service层
public interface ProductService {
List<Product> getAllProduct();
}
//service实现层
@Service
public class ProductServiceImpl implements ProductService {
@Resource
private ProductMapper productMapper;
@Override
public List<Product> getAllProduct() {
return productMapper.getAllProduct();
}
}
//mapper层
public interface ProductMapper {
List<Product> getAllProduct();
}
- ProductMapper接口实现层
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.web.mapper.ProductMapper">
<resultMap id="productMap" type="com.web.entity.Product">
<id column="id" property="id"/>
<result column="name" property="name"/>
<result column="pic" property="pic"/>
<result column="sale" property="sale"/>
<result column="price" property="price"/>
<result column="description" property="description"/>
<result column="stock" property="stock"/>
<result column="weight" property="weight"/>
<result column="brand_name" property="brandName"/>
</resultMap>
<select id="getAllProduct" parameterType="com.web.entity.Product" resultMap="productMap">
select * from product
</select>
</mapper>
三、FileController实现图片上传
package com.web.controller;
import java.io.File;
import org.apache.commons.io.FileUtils;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/file")
public class FileController {
@RequestMapping("/download")
public ResponseEntity<byte[]> download(String filename) {
// 设置编码
try {
String newFileName = new String(filename.getBytes("UTF-8"), "ISO-8859-1");
// 文件所在路径
String path = "D:\\";
// 文件下载的全路径
String downPath = path + filename;
// 生成下载的文件
File file = new File(downPath);
// 设置HttpHeaders,使得浏览器响应下载
HttpHeaders headers = new HttpHeaders();
// 给浏览器设置下载文件名
headers.setContentDispositionFormData("attachment", newFileName);
// 浏览器响应方式
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
// 把文件以二进制格式响应给浏览器
ResponseEntity<byte[]> responseEntity = new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),
headers, HttpStatus.OK);
return responseEntity;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
四、layUI和layUIMINI整合页面
导入layUI包和layUImini的lib包和css包到static目录下
新建productList.html,通过thymeleaf导入相关包
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" th:href="@{/layui/css/layui.css}" media="all">
<link rel="stylesheet" th:href="@{/css/layuimini.css?v=2.0.4.2}" media="all">
<link rel="stylesheet" th:href="@{/css/themes/default.css}" media="all">
<link rel="stylesheet" th:href="@{/lib/font-awesome-4.7.0/css/font-awesome.min.css}" media="all">
</head>
<body>
<script th:src="@{/lib/jquery-3.4.1/jquery-3.4.1.min.js}" charset="utf-8"></script>
<script th:src="@{/layui/layui.js}" charset="utf-8"></script>
</body>
</html>
整合layui数据表格
<table id="table-product"></table>
<script th:inline="javascript">
var ctxPath = [[@{/}]];
layui.use('table', function(){
var table = layui.table;
table.render({
elem: '#table-product'
,url: ctxPath + 'product' //数据接口
,limit: 15
,cols: [[ //表头,下面为表中个字段
{field: 'id', title: '编号', width:80, sort: true, align:'center'}
,{field: 'name', title: '商品名称', width:100, align:'center'}
,{field: 'pic', title: '图片', width:120, align:'center', templet:function (d) {
return '<img src="/file/download?filename='+d.pic+'"/>'}}
,{field: 'sale', title: '销量', width:80, sort: true, align:'center'}
,{field: 'price', title: '价格', width: 100, sort: true, align:'center'}
,{field: 'description', title: '描述', width: 200, align:'center'}
,{field: 'stock', title: '库存', width: 120, sort: true, align:'center'}
,{field: 'weight', title: '重量(kg)', width: 120, align:'center'}
,{field: 'brandName', title: '品牌', width: 150, align:'center'}
]]
});
});
</script>
展示图片重点在于:
templet:function (d) {return '<img src="/file/download?filename='+d.pic+'"/>'}
效果如下
图片展示不全,审查元素,找到展示图片所在的标签
然后对图片的样式自定义
<style type="text/css">
.layui-table-cell{
text-align:center;
height: auto;
white-space: normal;
}
.layui-table img{
max-width:100%
}
</style>
注意:单纯修改pic字段的width并不起作用,这里的width主要是控制表格列的宽度,高度不能通过height修改,所以需要按上面的方式改变图片样式,将图片完全展示出来。
最终product.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" th:href="@{/layui/css/layui.css}" media="all">
<link rel="stylesheet" th:href="@{/css/layuimini.css?v=2.0.4.2}" media="all">
<link rel="stylesheet" th:href="@{/css/themes/default.css}" media="all">
<link rel="stylesheet" th:href="@{/lib/font-awesome-4.7.0/css/font-awesome.min.css}" media="all">
</head>
<body>
<table id="table-product"></table>
<style type="text/css">
.layui-table-cell{
text-align:center;
height: auto;
white-space: normal;
}
.layui-table img{
max-width:100%
}
</style>
<script th:src="@{/lib/jquery-3.4.1/jquery-3.4.1.min.js}" charset="utf-8"></script>
<script th:src="@{/layui/layui.js}" charset="utf-8"></script>
<script th:inline="javascript">
var ctxPath = [[@{/}]];
layui.use('table', function(){
var table = layui.table;
table.render({
elem: '#table-product'
,url: ctxPath + 'product' //数据接口
,limit: 15
,cols: [[ //表头,下面为表中个字段
{field: 'id', title: '编号', width:80, sort: true, align:'center'}
,{field: 'name', title: '商品名称', width:100, align:'center'}
,{field: 'pic', title: '图片', width:120, align:'center', templet:function (d) {
return '<img src="/file/download?filename='+d.pic+'"/>'}}
,{field: 'sale', title: '销量', width:80, sort: true, align:'center'}
,{field: 'price', title: '价格', width: 100, sort: true, align:'center'}
,{field: 'description', title: '描述', width: 200, align:'center'}
,{field: 'stock', title: '库存', width: 120, sort: true, align:'center'}
,{field: 'weight', title: '重量(kg)', width: 120, align:'center'}
,{field: 'brandName', title: '品牌', width: 150, align:'center'}
]]
});
});
</script>
</body>
</html>
最后展示样式
更多推荐
所有评论(0)