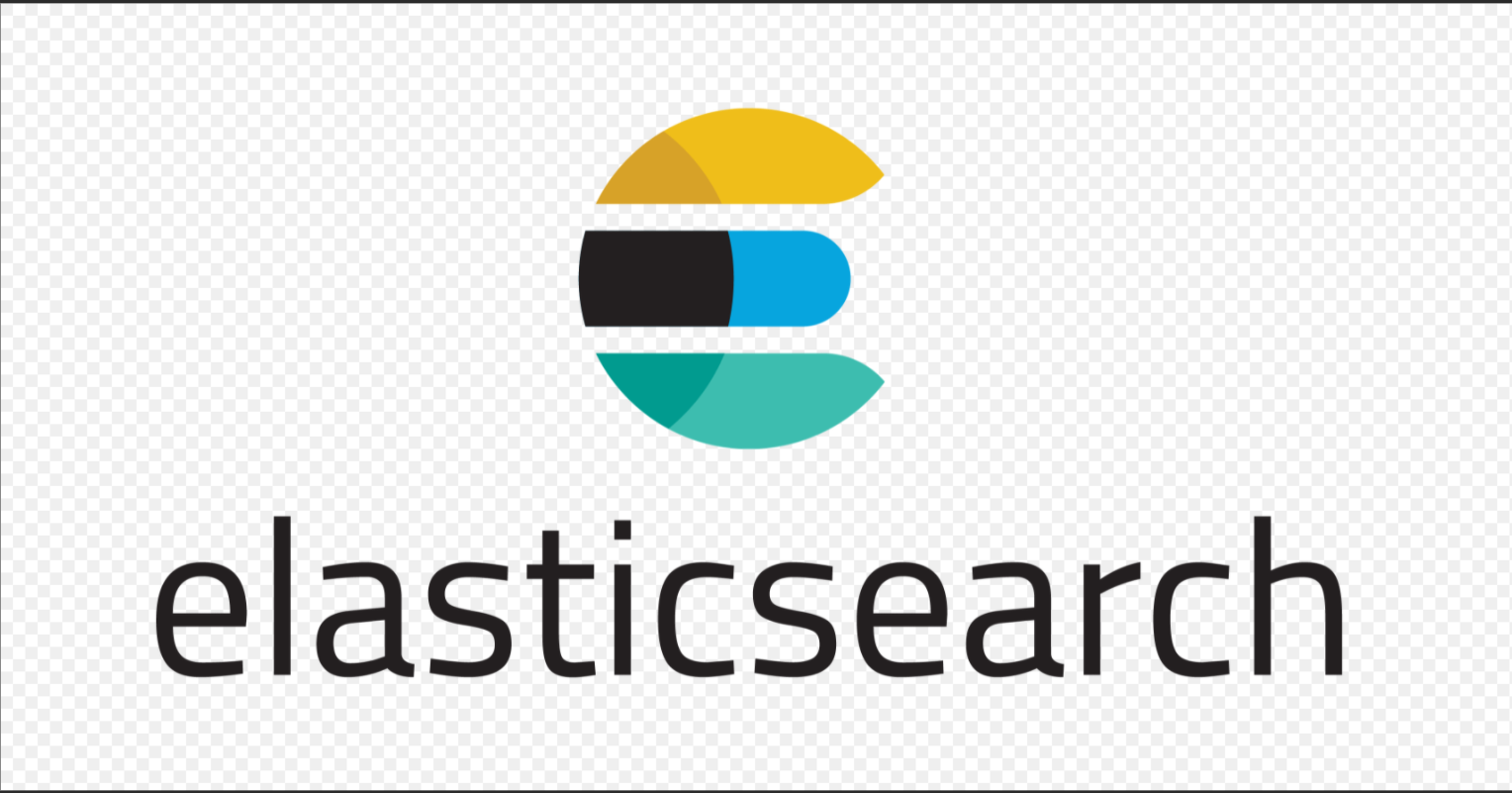
最详细的java 代码 操作es数据库
java代码操作es,内容最详细,最容易理解,最容易上手。
1:提前准备环境以及es软件 ,大家可以去官网下载,我用的是es window 7.4的
es官网:https://www.elastic.co/cn/downloads/elasticsearch
<dependencies>
<dependency>
<groupId>org.elasticsearch</groupId>
<artifactId>elasticsearch</artifactId>
<version>7.8.0</version>
</dependency>
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.8.0</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.8.2</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.8.2</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.9</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
</dependencies>
2:然后打开idea创建好准备好,请看下面代码,着里面的代码如果大家看过我之前写过的postMan的创建索引库的方式其实是差不多的.
public class esTest {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost",9200,"http"))
);
// 创建索引库名称
CreateIndexRequest request = new CreateIndexRequest("qianyuedong");
// 创建索引库
CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT);
// 打印是否创建成功
System.out.println("操作索引库"+response.isAcknowledged());
// 关闭客户端
client.close();
}
}
3:请看返回结果
这时候我们可以去用postman去查看索引库的信息 localhost:9200/_cat/indices?v
2:接下来我们来查询刚刚所创建的索引库
(1):其实大部分代码都是一样的。大家只有稍微的加以理解很快就能明白其中的道理下面请看例子
public class esTestRequest {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost",9200,"http"))
);
//查询索引库
GetIndexRequest request = new GetIndexRequest("qian");
GetIndexResponse response = client.indices().get(request, RequestOptions.DEFAULT);
// 查看查询结果
System.out.println(response.getAliases());
System.out.println(response.getMappings());
System.out.println(response.getSettings());
// 关闭客户端
client.close();
}
}
这里面的打印结果跟我们之前postMan查询的结果是一样的,相信大家看到这里大概就能明白了。这里就不多做解释了。如果大家还是不明白去看看我之前写的
“es的基本操作”
“和es多条件查询”
3:删除索引库,其实大家看到了这里面大家都明白了是怎么一回事了
public class esTestDelRequest {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost",9200,"http"))
);
//删除索引库
DeleteIndexRequest request = new DeleteIndexRequest("qian");
AcknowledgedResponse response = client.indices().delete(request, RequestOptions.DEFAULT);
// 查看查询结果
boolean acknowledged = response.isAcknowledged();
System.out.println("删除索引库成功"+acknowledged);
// 关闭客户端
client.close();
}
执行结果:
之前我们postman的结果
4:增加文档数据
上面我们把索引库已经的一些基本的创建查询已经全部用java 代码所实现了,下面我们把数据 加入到 索引库中,但是要注意的是,再我们创建索引库的时候用的客户端的indices中的,但是再创建数据的时候我们需要用index下面请看例子,
准备 一个对象,这个对象方便我们下次把数据转成json格式存入index数据库中。
import lombok.Data;
@Data
class Person {
private String name;
private String sex;
private String tel;
}
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.security.user.User;
import org.elasticsearch.common.xcontent.XContentType;
import java.io.IOException;
public class esInsert {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(new HttpHost("localhost", 9200, "http")));
//插入数据,再前面我们都是创建indices然后去创建跟索引库相关的
IndexRequest request = new IndexRequest();
// 插入数据
request.index("qian").id("1001");
//准备数据因为再ES中都是json格式的数据所以我们前期准备的依赖也准备fastjson依赖
Person person = new Person();
person.setName("张三");
person.setSex("男的");
person.setTel("17721650570");
// String string = JSON.toJSONString(person);
// 或者这样装成json
ObjectMapper mapper = new ObjectMapper();
String jsonPerson = mapper.writeValueAsString(person);
// 再将我们的json数据放进request中作为数据源
request.source(jsonPerson, XContentType.JSON);
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
// 查看结果
DocWriteResponse.Result result = response.getResult();
// 关闭客户端
System.out.println(request);
client.close();
}
}
IDEA控制台打印的结果
Connected to the target VM, address: '127.0.0.1:60845', transport: 'socket'
index {[qian][_doc][1001], source[{"name":"张三","sex":"男的","tel":"17721650570"}]}
Disconnected from the target VM, address: '127.0.0.1:60845', transport: 'socket'
Process finished with exit code 0
我们在回忆一下,之前我们用postman客户端,再索引库中 打印的结果把
5:修改文档数据
修改索引库中的数据源,修改的时候我们操作的是文档数据,doc,再es中之前也跟大家说过文档数据再是es的核心。下面请看用java代码如何实现修改es中的数据
大家请先看一下之前我们用postman工具修改文档的数据。
估计大家对文档数据据的修改也有了大概的了解,下面请大家看一下,java代码是如何修改数据的。
public class esUapate {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(new HttpHost("localhost", 9200, "http")));
//插入数据,再前面我们都是创建indices然后去创建跟索引库相关的
UpdateRequest request = new UpdateRequest();
//在修改数据的时候我们就不叫index,应该是update
// 修改数据我们是对文档的数据进行修改
request.index("qian").id("1001"); // 表示对哪个索引库进行修改对那条数据进行修改
// doc 表示文档数据
request.doc(XContentType.JSON,"sex","女");
UpdateResponse response = client.update(request, RequestOptions.DEFAULT);
// 打印结果
System.out.println(response.getResult());
client.close();
}
}
控制台结果然后我们去postman工具查看是否修改成功
相信大家看到这里对es的基本数据的操作用java代码也有个基本的认识如果还有不明白的去es官网,官网上的例子也很直白。
6:文档数据的查询
public class EsDOC_GetRequest {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(new HttpHost("localhost", 9200, "http")));
// 所有的 方式都是要拿到查询的条件,大家可以看之间所有写过的东西
GetRequest request = new GetRequest();
// 我们把我们要查询的条件放进去 查询的索引库以及查询数据id
request.index("qian").id("1001");
// 再构建完查询条件以后我们需要把条件放进去,所有对文档的操作大家都看到了用客户端client.index去完成
// 之前我们再创建索引库的时候用的是client.indices.create或者delete ,get。
GetResponse response = client.get(request, RequestOptions.DEFAULT);
// 打印结果都是跟数据源相关的,这俩中写法都行
System.out.println(response.getSourceAsString());
// System.out.println(response.getSourceAsMap());
client.close();
}
}
查看打印结果j:
这就是我们的查询文档数据,大家觉得是不是很简单。
7:文档数据的删除
public class EsDOC_Delete {
public static void main(String[] args) throws IOException {
// 创建es客户端
RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(new HttpHost("localhost", 9200, "http")));
// 删除索引库,首先还是创建删除的条件
DeleteRequest request = new DeleteRequest();
// 构建删除的索引库名称以及删除索引库的文档数据是哪一条
request.index("qian").id("1001");
DeleteResponse response = client.delete(request, RequestOptions.DEFAULT);
//打印结果
System.out.println(response.getResult());
System.out.println(response.toString());
client.close();
}
}
查看打印结果
倒这里我们的文档数据的curd和索引库的curd到这里就结束了大家看起来是不是还是很简单的今天本章用java代码的简单索引库的创建和简单的数据增修改,就到这里了,下一篇我们继续学习,和聚合查询,高亮查询。。。。继续,喜欢的请关注,转发 收藏,感谢。
更多推荐
所有评论(0)